java hmacsha1 密钥加密
时间: 2023-07-29 10:13:40 浏览: 114
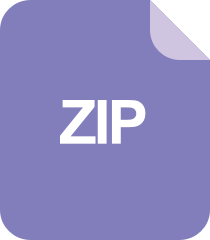
HMAC_hmacsha_
您可以使用以下代码片段在 Java 中使用 HMAC-SHA1 对数据进行密钥加密:
```java
import javax.crypto.Mac;
import javax.crypto.spec.SecretKeySpec;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
public class HmacSha1Encryption {
public static void main(String[] args) {
String data = "Hello World!";
String key = "my-secret-key";
try {
byte[] encryptedData = hmacSha1(data, key);
System.out.println("Encrypted data: " + bytesToHex(encryptedData));
} catch (NoSuchAlgorithmException | InvalidKeyException e) {
e.printStackTrace();
}
}
public static byte[] hmacSha1(String data, String key)
throws NoSuchAlgorithmException, InvalidKeyException {
String algorithm = "HmacSHA1";
Mac mac = Mac.getInstance(algorithm);
SecretKeySpec keySpec = new SecretKeySpec(key.getBytes(), algorithm);
mac.init(keySpec);
return mac.doFinal(data.getBytes());
}
private static final char[] HEX_ARRAY = "0123456789ABCDEF".toCharArray();
public static String bytesToHex(byte[] bytes) {
char[] hexChars = new char[bytes.length * 2];
for (int i = 0; i < bytes.length; i++) {
int v = bytes[i] & 0xFF;
hexChars[i * 2] = HEX_ARRAY[v >>> 4];
hexChars[i * 2 + 1] = HEX_ARRAY[v & 0x0F];
}
return new String(hexChars);
}
}
```
请注意,这里使用的密钥是字符串形式的。在实际应用中,您应该将密钥存储为一个 byte 数组,并使用 `SecretKeySpec` 类来创建密钥规范。
阅读全文
相关推荐
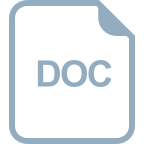
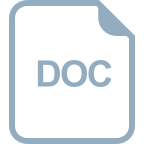
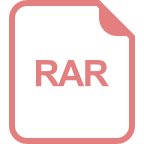


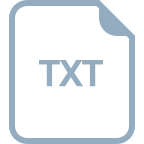
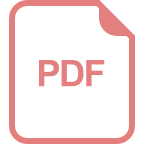
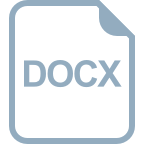
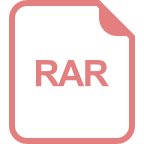








