java springboot发起微信jsapi支付 使用v2密钥具体代码怎么编写
时间: 2024-06-11 09:08:42 浏览: 11
下面是Java SpringBoot发起微信JSAPI支付使用V2密钥的代码示例:
1. 首先,在SpringBoot的配置文件中添加微信支付的相关配置信息:
```yaml
wxpay:
app-id: YOUR_APP_ID
mch-id: YOUR_MCH_ID
v2-key: YOUR_V2_KEY
notify-url: YOUR_NOTIFY_URL
```
2. 然后,在SpringBoot中定义微信支付的服务类:
```java
@Service
public class WxPayService {
@Autowired
private WxPayProperties wxPayProperties;
public Map<String, String> createOrder(String openid, String outTradeNo, int totalFee, String body, String ipAddress) throws Exception {
// 构造请求参数
Map<String, String> data = new HashMap<>();
data.put("appid", wxPayProperties.getAppId());
data.put("mch_id", wxPayProperties.getMchId());
data.put("nonce_str", UUID.randomUUID().toString().replaceAll("-", ""));
data.put("body", body);
data.put("out_trade_no", outTradeNo);
data.put("total_fee", String.valueOf(totalFee));
data.put("spbill_create_ip", ipAddress);
data.put("notify_url", wxPayProperties.getNotifyUrl());
data.put("trade_type", "JSAPI");
data.put("openid", openid);
// 生成签名
String sign = WxPayUtil.generateSignature(data, wxPayProperties.getV2Key());
data.put("sign", sign);
// 发起统一下单请求
String unifiedOrderUrl = "https://api.mch.weixin.qq.com/pay/unifiedorder";
String result = HttpUtil.post(unifiedOrderUrl, WxPayUtil.toXml(data));
Map<String, String> resultMap = WxPayUtil.xmlToMap(result);
// 判断下单是否成功
if ("SUCCESS".equals(resultMap.get("return_code")) && "SUCCESS".equals(resultMap.get("result_code"))) {
// 构造JSAPI支付参数
Map<String, String> payData = new HashMap<>();
payData.put("appId", wxPayProperties.getAppId());
payData.put("timeStamp", String.valueOf(System.currentTimeMillis() / 1000));
payData.put("nonceStr", UUID.randomUUID().toString().replaceAll("-", ""));
payData.put("package", "prepay_id=" + resultMap.get("prepay_id"));
payData.put("signType", "MD5");
// 生成签名
String paySign = WxPayUtil.generateSignature(payData, wxPayProperties.getV2Key());
payData.put("paySign", paySign);
return payData;
} else {
throw new Exception(resultMap.get("return_msg"));
}
}
}
```
3. 最后,在Controller层调用微信支付服务类:
```java
@RestController
@RequestMapping("/wxpay")
public class WxPayController {
@Autowired
private WxPayService wxPayService;
@PostMapping("/createOrder")
public Map<String, String> createOrder(@RequestParam String openid, @RequestParam String outTradeNo, @RequestParam int totalFee, @RequestParam String body, HttpServletRequest request) throws Exception {
String ipAddress = HttpUtil.getIpAddress(request);
return wxPayService.createOrder(openid, outTradeNo, totalFee, body, ipAddress);
}
}
```
其中,HttpUtil是一个工具类,用于发送HTTP请求。WxPayUtil是一个微信支付的工具类,用于生成签名、XML转换等操作。以上代码示例中,仅提供了微信支付的主要逻辑。具体实现中,还需要根据实际情况,添加异常处理、日志记录等功能。
相关推荐
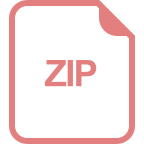
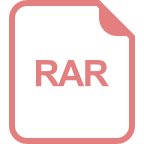










