用c语言功能要求: (1) 输入一个年份,输出是在屏幕上显示该年的日历。假定输入的年份在1940-2040年之间。 (2) 输入年月,输出该月的日历。 (3) 输入年月日,输出距今天还有多少天,星期几,是否是公历节日。
时间: 2024-03-30 11:38:47 浏览: 88
好的,以下是用C语言实现你提出的三个功能要求的代码示例:
1. 输入一个年份,输出是在屏幕上显示该年的日历
```c
#include <stdio.h>
// 判断是否是闰年
int isLeapYear(int year) {
return (year % 4 == 0 && year % 100 != 0) || year % 400 == 0;
}
// 获取某个月的天数
int getDaysOfMonth(int year, int month) {
int days[] = {31, 28 + isLeapYear(year), 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
return days[month - 1];
}
// 输出某一年的日历
void printCalendarOfYear(int year) {
printf("%d年的日历:\n", year);
int i, j, days, weekday = 0;
char *monthNames[] = {"一月", "二月", "三月", "四月", "五月", "六月", "七月", "八月", "九月", "十月", "十一月", "十二月"};
for (i = 1; i <= 12; i++) {
printf("\n%s\n", monthNames[i - 1]);
printf("--------------------\n");
printf("日 一 二 三 四 五 六\n");
days = getDaysOfMonth(year, i);
for (j = 1; j <= days; j++) {
if (j == 1) {
weekday = i == 1 ? year % 7 : (weekday + getDaysOfMonth(year, i - 1)) % 7;
while (weekday--) {
printf(" ");
}
}
printf("%2d ", j);
weekday = (weekday + 1) % 7;
if (weekday == 0) {
printf("\n");
}
}
if (weekday != 0) {
printf("\n");
}
}
}
int main() {
int year;
printf("请输入年份(1940-2040):");
scanf("%d", &year);
if (year >= 1940 && year <= 2040) {
printCalendarOfYear(year);
} else {
printf("输入的年份无效!");
}
return 0;
}
```
2. 输入年月,输出该月的日历。
```c
#include <stdio.h>
// 判断是否是闰年
int isLeapYear(int year) {
return (year % 4 == 0 && year % 100 != 0) || year % 400 == 0;
}
// 获取某个月的天数
int getDaysOfMonth(int year, int month) {
int days[] = {31, 28 + isLeapYear(year), 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
return days[month - 1];
}
// 输出某个月的日历
void printCalendarOfMonth(int year, int month) {
printf("%d年%d月的日历:\n", year, month);
printf("--------------------\n");
printf("日 一 二 三 四 五 六\n");
int i, days, weekday = 0;
days = getDaysOfMonth(year, month);
for (i = 1; i <= days; i++) {
if (i == 1) {
weekday = (getDaysOfMonth(year, month - 1) + 1) % 7;
while (weekday--) {
printf(" ");
}
}
printf("%2d ", i);
weekday = (weekday + 1) % 7;
if (weekday == 0) {
printf("\n");
}
}
if (weekday != 0) {
printf("\n");
}
}
int main() {
int year, month;
printf("请输入年份(1940-2040)和月份(1-12):");
scanf("%d%d", &year, &month);
if (year >= 1940 && year <= 2040 && month >= 1 && month <= 12) {
printCalendarOfMonth(year, month);
} else {
printf("输入的年份或月份无效!");
}
return 0;
}
```
3. 输入年月日,输出距今天还有多少天,星期几,是否是公历节日。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
// 判断是否是闰年
int isLeapYear(int year) {
return (year % 4 == 0 && year % 100 != 0) || year % 400 == 0;
}
// 获取某个月的天数
int getDaysOfMonth(int year, int month) {
int days[] = {31, 28 + isLeapYear(year), 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
return days[month - 1];
}
// 判断是否是公历节日
int isSolarFestival(int month, int day, char *festival) {
char *solarFestivals[] = {"元旦", "情人节", "妇女节", "植树节", "劳动节", "青年节", "儿童节", "建党节", "建军节", "教师节", "国庆节", "平安夜", "圣诞节"};
int solarDays[] = {1, 14, 8, 12, 1, 4, 1, 1, 1, 10, 1, 24, 25};
int solarMonths[] = {1, 2, 3, 3, 5, 5, 6, 7, 8, 9, 10, 12, 12};
int i;
for (i = 0; i < 13; i++) {
if (month == solarMonths[i] && day == solarDays[i]) {
strcpy(festival, solarFestivals[i]);
return 1;
}
}
return 0;
}
// 获取当前日期
void getCurrentDate(int *year, int *month, int *day) {
time_t t = time(NULL);
struct tm *tm = localtime(&t);
*year = tm->tm_year + 1900;
*month = tm->tm_mon + 1;
*day = tm->tm_mday;
}
// 计算指定日期距今天还有多少天
int getDaysToToday(int year, int month, int day) {
int i, days = 0;
int daysOfCurMonth = getDaysOfMonth(year, month);
for (i = day + 1; i <= daysOfCurMonth; i++) {
days++;
}
for (i = month + 1; i <= 12; i++) {
days += getDaysOfMonth(year, i);
}
for (i = year + 1; i < 2041; i++) {
days += isLeapYear(i) ? 366 : 365;
}
return days;
}
// 计算指定日期是星期几
int getWeekday(int year, int month, int day) {
int days = 0, i;
for (i = 1940; i < year; i++) {
days += isLeapYear(i) ? 366 : 365;
}
for (i = 1; i < month; i++) {
days += getDaysOfMonth(year, i);
}
days += day;
return days % 7;
}
int main() {
int year, month, day, daysToToday, weekday;
char festival[10] = "";
getCurrentDate(&year, &month, &day);
printf("今天是%d年%d月%d日\n", year, month, day);
printf("请输入一个日期(1940-2040):");
scanf("%d%d%d", &year, &month, &day);
if (year >= 1940 && year <= 2040 && month >= 1 && month <= 12 && day >= 1 && day <= getDaysOfMonth(year, month)) {
daysToToday = getDaysToToday(year, month, day);
weekday = getWeekday(year, month, day);
if (isSolarFestival(month, day, festival)) {
printf("距离今天还有%d天,星期%d,是公历节日:%s", daysToToday, weekday, festival);
} else {
printf("距离今天还有%d天,星期%d", daysToToday, weekday);
}
} else {
printf("输入的日期无效!");
}
return 0;
}
```
希望这些代码能够帮助你实现你的功能要求。
阅读全文
相关推荐
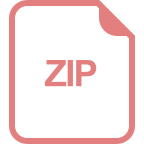




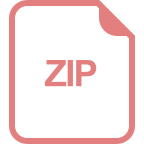
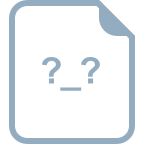
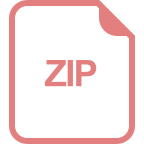



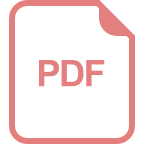