单色位图抗锯齿算法MFC代码实现
时间: 2023-10-22 08:20:38 浏览: 50
以下是单色位图抗锯齿算法的MFC代码实现:
```c++
void AntiAlias(CDC* pDC, CBitmap* pBitmap)
{
// 获取位图信息
BITMAP bmpInfo;
pBitmap->GetBitmap(&bmpInfo);
// 创建内存DC和位图
CDC dcMem;
dcMem.CreateCompatibleDC(pDC);
CBitmap bmpMem;
bmpMem.CreateCompatibleBitmap(pDC, bmpInfo.bmWidth, bmpInfo.bmHeight);
CBitmap* pOldBmp = dcMem.SelectObject(&bmpMem);
// 将位图绘制到内存DC中
dcMem.BitBlt(0, 0, bmpInfo.bmWidth, bmpInfo.bmHeight, pDC, 0, 0, SRCCOPY);
// 获取位图的像素数据
BYTE* pBits = new BYTE[bmpInfo.bmWidth * bmpInfo.bmHeight];
::ZeroMemory(pBits, bmpInfo.bmWidth * bmpInfo.bmHeight);
::GetBitmapBits(pBitmap->m_hBitmap, bmpInfo.bmWidth * bmpInfo.bmHeight, pBits);
// 对每个像素进行抗锯齿处理
for (int y = 0; y < bmpInfo.bmHeight; y++)
{
for (int x = 0; x < bmpInfo.bmWidth; x++)
{
BYTE* pPixel = pBits + y * bmpInfo.bmWidth + x;
if (*pPixel == 0) // 如果当前像素是黑色,则需要进行抗锯齿处理
{
// 获取当前像素周围8个像素的颜色值
BYTE color[8];
::ZeroMemory(color, sizeof(color));
if (x > 0 && y > 0)
color[0] = *(pBits + (y - 1) * bmpInfo.bmWidth + x - 1);
if (y > 0)
color[1] = *(pBits + (y - 1) * bmpInfo.bmWidth + x);
if (x < bmpInfo.bmWidth - 1 && y > 0)
color[2] = *(pBits + (y - 1) * bmpInfo.bmWidth + x + 1);
if (x > 0)
color[3] = *(pBits + y * bmpInfo.bmWidth + x - 1);
if (x < bmpInfo.bmWidth - 1)
color[4] = *(pBits + y * bmpInfo.bmWidth + x + 1);
if (x > 0 && y < bmpInfo.bmHeight - 1)
color[5] = *(pBits + (y + 1) * bmpInfo.bmWidth + x - 1);
if (y < bmpInfo.bmHeight - 1)
color[6] = *(pBits + (y + 1) * bmpInfo.bmWidth + x);
if (x < bmpInfo.bmWidth - 1 && y < bmpInfo.bmHeight - 1)
color[7] = *(pBits + (y + 1) * bmpInfo.bmWidth + x + 1);
// 计算当前像素的平均颜色值
BYTE avgColor = 0;
int count = 0;
for (int i = 0; i < 8; i++)
{
if (color[i] > 0)
{
avgColor += color[i];
count++;
}
}
if (count > 0)
avgColor /= count;
// 将当前像素的颜色值设为平均颜色值
*pPixel = avgColor;
}
}
}
// 将处理后的位图绘制到屏幕上
pDC->BitBlt(0, 0, bmpInfo.bmWidth, bmpInfo.bmHeight, &dcMem, 0, 0, SRCCOPY);
// 释放资源
delete[] pBits;
dcMem.SelectObject(pOldBmp);
bmpMem.DeleteObject();
dcMem.DeleteDC();
}
```
使用方式:
```c++
CBitmap bmp;
bmp.LoadBitmap(IDB_BITMAP1);
AntiAlias(pDC, &bmp);
```
相关推荐
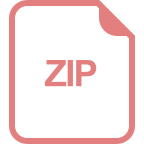










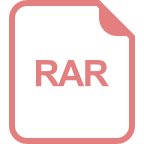
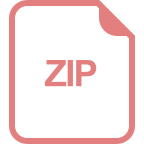