如何配置websocket在springboot
时间: 2023-07-05 21:10:44 浏览: 58
在 Spring Boot 中配置 WebSocket 需要以下步骤:
1. 添加 WebSocket 相关依赖。在 pom.xml 文件中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-websocket</artifactId>
</dependency>
```
2. 创建 WebSocket 配置类。创建一个 WebSocketConfig 类,并添加 @Configuration 和 @EnableWebSocket 注解。
```java
import org.springframework.context.annotation.Configuration;
import org.springframework.web.socket.config.annotation.EnableWebSocket;
import org.springframework.web.socket.config.annotation.WebSocketConfigurer;
import org.springframework.web.socket.config.annotation.WebSocketHandlerRegistry;
@Configuration
@EnableWebSocket
public class WebSocketConfig implements WebSocketConfigurer {
@Override
public void registerWebSocketHandlers(WebSocketHandlerRegistry registry) {
registry.addHandler(new MyWebSocketHandler(), "/my-websocket")
.setAllowedOrigins("*"); // 允许跨域
}
}
```
3. 创建 WebSocket 处理器。创建一个 WebSocket 处理器类,实现 WebSocketHandler 接口,并重写相关方法。
```java
import org.springframework.web.socket.CloseStatus;
import org.springframework.web.socket.TextMessage;
import org.springframework.web.socket.WebSocketHandler;
import org.springframework.web.socket.WebSocketMessage;
import org.springframework.web.socket.WebSocketSession;
public class MyWebSocketHandler implements WebSocketHandler {
@Override
public void afterConnectionEstablished(WebSocketSession session) throws Exception {
// 连接建立时执行
}
@Override
public void handleMessage(WebSocketSession session, WebSocketMessage<?> message) throws Exception {
// 处理消息时执行
}
@Override
public void handleTransportError(WebSocketSession session, Throwable exception) throws Exception {
// 发生错误时执行
}
@Override
public void afterConnectionClosed(WebSocketSession session, CloseStatus closeStatus) throws Exception {
// 连接关闭时执行
}
@Override
public boolean supportsPartialMessages() {
return false;
}
}
```
4. 发送消息。在需要发送消息的地方,通过 WebSocketSession 对象发送消息。
```java
session.sendMessage(new TextMessage("Hello, WebSocket!"));
```
以上是在 Spring Boot 中配置 WebSocket 的基本步骤,具体实现还需要根据业务需求进行调整。
相关推荐
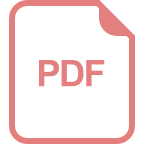
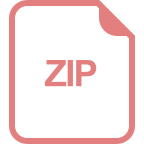
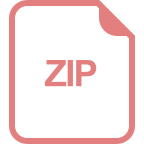














