用python代码实现使用KNN算法识别mnist.test_image中的每个图片对应的数值并于mnist.test_labels进行比较看是否正确。已有mnist.train_image和mnist.test_labels
时间: 2024-05-01 20:20:04 浏览: 14
首先,需要导入必要的库和数据集:
```python
import numpy as np
from sklearn.neighbors import KNeighborsClassifier
from tensorflow.examples.tutorials.mnist import input_data
mnist = input_data.read_data_sets("MNIST_data/", one_hot=False)
```
然后,将训练集和测试集的图像数据和标签提取出来:
```python
train_images = mnist.train.images
train_labels = mnist.train.labels
test_images = mnist.test.images
test_labels = mnist.test.labels
```
接下来,我们可以使用KNN算法进行训练和预测:
```python
knn = KNeighborsClassifier(n_neighbors=3)
knn.fit(train_images, train_labels)
predicted_labels = knn.predict(test_images)
```
最后,我们可以比较预测的标签和测试集中的真实标签,计算模型的准确率:
```python
accuracy = np.mean(predicted_labels == test_labels)
print("Accuracy:", accuracy)
```
完整的代码如下:
```python
import numpy as np
from sklearn.neighbors import KNeighborsClassifier
from tensorflow.examples.tutorials.mnist import input_data
mnist = input_data.read_data_sets("MNIST_data/", one_hot=False)
train_images = mnist.train.images
train_labels = mnist.train.labels
test_images = mnist.test.images
test_labels = mnist.test.labels
knn = KNeighborsClassifier(n_neighbors=3)
knn.fit(train_images, train_labels)
predicted_labels = knn.predict(test_images)
accuracy = np.mean(predicted_labels == test_labels)
print("Accuracy:", accuracy)
```
相关推荐
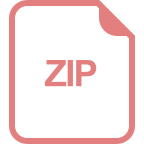













