用c语言写一个银行排队模型代码
时间: 2023-05-14 14:05:41 浏览: 221
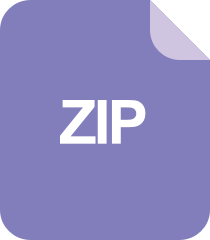
C语言编写的银行排队系统

以下是一个简单的银行排队模型的 C 语言代码:
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define MAX_TIME 100 // 模拟时间
#define ARRIVAL_INTERVAL 5 // 客户到达时间间隔
#define SERVICE_TIME 10 // 客户服务时间
typedef struct {
int arrival_time; // 到达时间
int service_time; // 服务时间
int start_time; // 开始服务时间
int end_time; // 结束服务时间
} Customer;
typedef struct {
Customer *queue; // 队列
int front; // 队头
int rear; // 队尾
int size; // 队列大小
} Queue;
void init_queue(Queue *q, int size) {
q->queue = (Customer *)malloc(sizeof(Customer) * size);
q->front = q->rear = 0;
q->size = size;
}
void enqueue(Queue *q, Customer c) {
if ((q->rear + 1) % q->size == q->front) {
printf("Queue is full.\n");
return;
}
q->queue[q->rear] = c;
q->rear = (q->rear + 1) % q->size;
}
Customer dequeue(Queue *q) {
if (q->front == q->rear) {
printf("Queue is empty.\n");
return (Customer){0, 0, 0, 0};
}
Customer c = q->queue[q->front];
q->front = (q->front + 1) % q->size;
return c;
}
int is_empty(Queue *q) {
return q->front == q->rear;
}
int main() {
srand(time(NULL));
Queue q;
init_queue(&q, 10);
int current_time = 0;
while (current_time < MAX_TIME) {
if (rand() % ARRIVAL_INTERVAL == 0) {
Customer c = {current_time, SERVICE_TIME, 0, 0};
enqueue(&q, c);
printf("Customer arrived at %d.\n", current_time);
}
if (!is_empty(&q)) {
Customer c = dequeue(&q);
if (c.start_time == 0) {
c.start_time = current_time;
}
c.service_time--;
if (c.service_time == 0) {
c.end_time = current_time;
printf("Customer served from %d to %d.\n", c.start_time, c.end_time);
} else {
enqueue(&q, c);
}
}
current_time++;
}
return 0;
}
阅读全文
相关推荐
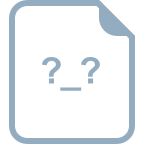

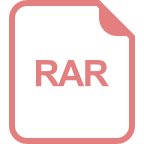

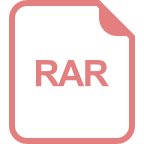
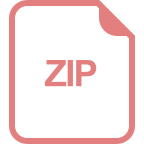
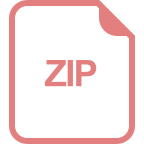
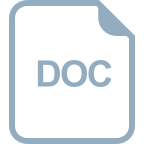
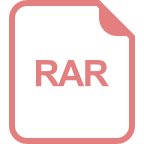
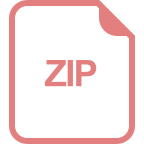
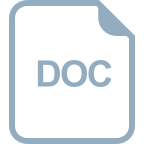
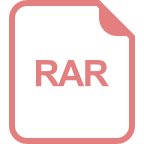
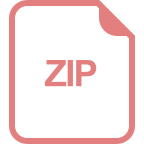
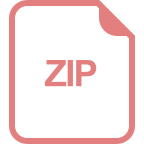
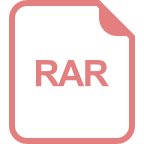
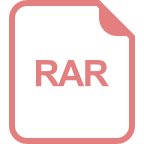
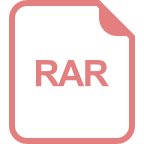