c++单链表火车信息
时间: 2023-07-04 19:25:49 浏览: 60
好的,我可以帮你讲解一下如何使用C++单链表来存储火车信息。
首先需要定义一个火车信息的结构体,例如:
```c++
struct Train {
string name; // 火车名
string from; // 起点站
string to; // 终点站
string time; // 发车时间
};
```
接下来,定义一个单链表节点的结构体,其中包含一个火车信息的结构体和一个指向下一个节点的指针,例如:
```c++
struct Node {
Train train; // 火车信息
Node* next; // 指向下一个节点的指针
};
```
定义一个单链表的类,其中包含头节点指针和尾节点指针,以及一些操作方法,例如:
```c++
class TrainList {
public:
TrainList() {
head = new Node();
tail = head;
}
~TrainList() {
Node* current = head;
while (current != nullptr) {
Node* tmp = current;
current = current->next;
delete tmp;
}
}
void insert(const Train& train) {
Node* node = new Node();
node->train = train;
tail->next = node;
tail = node;
}
void remove(const Train& train) {
Node* current = head->next;
Node* prev = head;
while (current != nullptr) {
if (current->train.name == train.name &&
current->train.from == train.from &&
current->train.to == train.to &&
current->train.time == train.time) {
prev->next = current->next;
if (current == tail) {
tail = prev;
}
delete current;
break;
}
prev = current;
current = current->next;
}
}
void print() const {
Node* current = head->next;
while (current != nullptr) {
cout << "火车名:" << current->train.name << endl;
cout << "起点站:" << current->train.from << endl;
cout << "终点站:" << current->train.to << endl;
cout << "发车时间:" << current->train.time << endl;
cout << endl;
current = current->next;
}
}
private:
Node* head;
Node* tail;
};
```
然后在主函数中,可以创建一个TrainList对象,调用insert方法添加火车信息,调用remove方法删除火车信息,调用print方法打印所有火车信息,例如:
```c++
int main() {
TrainList trainList;
Train train1 = {"G1", "北京", "上海", "08:00"};
Train train2 = {"G2", "上海", "北京", "14:00"};
Train train3 = {"D1", "北京", "天津", "09:00"};
trainList.insert(train1);
trainList.insert(train2);
trainList.insert(train3);
trainList.print();
trainList.remove(train1);
trainList.print();
return 0;
}
```
以上就是使用C++单链表存储火车信息的示例,希望对你有所帮助。
相关推荐
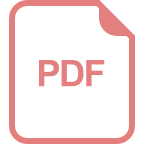
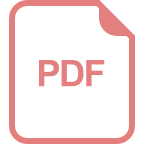














