qt中如何旋转按键
时间: 2023-10-05 07:07:23 浏览: 34
在Qt中,您可以使用QPropertyAnimation类来旋转按键。QPropertyAnimation类提供了一种简单的方式,用于在指定的时间内,从一个属性的当前值平滑地过渡到另一个属性的值。
以下是一个示例,演示如何旋转按键:
```cpp
class MyButton : public QPushButton
{
Q_OBJECT
public:
MyButton(QWidget *parent = 0);
public slots:
void rotate();
private:
QPropertyAnimation *m_animation;
};
MyButton::MyButton(QWidget *parent)
: QPushButton("Click me", parent)
{
// 创建旋转动画
m_animation = new QPropertyAnimation(this, "rotation");
m_animation->setDuration(1000);
m_animation->setStartValue(0);
m_animation->setEndValue(360);
m_animation->setLoopCount(-1); // 无限循环
// 连接按键的clicked()信号到槽函数rotate()
connect(this, SIGNAL(clicked()), this, SLOT(rotate()));
}
void MyButton::rotate()
{
// 启动旋转动画
m_animation->start();
}
```
在这个示例中,我们创建了一个名为MyButton的QPushButton派生类,并在其中添加了一个QPropertyAnimation对象。我们在构造函数中设置了旋转动画的持续时间、起始值和结束值,并将其循环次数设置为无限。我们还连接了按键的clicked()信号到槽函数rotate(),在该函数中启动旋转动画。
要使动画生效,我们需要添加一个rotation属性到MyButton类中。我们可以通过添加下面代码来实现:
```cpp
class MyButton : public QPushButton
{
Q_OBJECT
Q_PROPERTY(qreal rotation READ rotation WRITE setRotation)
public:
MyButton(QWidget *parent = 0);
qreal rotation() const { return m_rotation; }
void setRotation(qreal rotation) { m_rotation = rotation; update(); }
protected:
void paintEvent(QPaintEvent *event);
private:
qreal m_rotation;
};
void MyButton::paintEvent(QPaintEvent *event)
{
Q_UNUSED(event);
// 旋转绘图上下文并绘制按键
QPainter painter(this);
painter.translate(width() / 2, height() / 2);
painter.rotate(m_rotation);
painter.translate(-width() / 2, -height() / 2);
painter.drawText(rect(), Qt::AlignCenter, text());
}
```
在这里,我们添加了一个名为rotation的属性,并在paintEvent函数中使用QPainter来旋转绘图上下文并绘制按键。由于我们已经在MyButton类中添加了一个rotation属性,QPropertyAnimation将会自动更新该属性的值,并启动动画。
最后,您可以使用MyButton类来创建一个旋转的按键,例如:
```cpp
MyButton *button = new MyButton(this);
button->setGeometry(QRect(QPoint(100, 100), QSize(100, 50)));
```
请注意,这只是一个简单的示例,您可以根据需要自定义MyButton类,并使用更复杂的绘图算法来绘制旋转的按键。
阅读全文
相关推荐
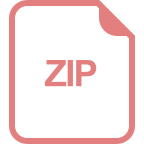
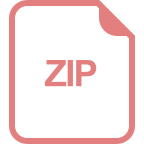
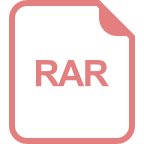
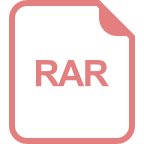
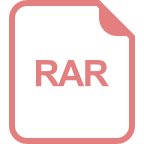
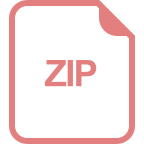
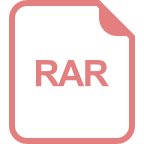
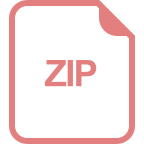
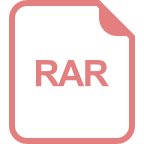
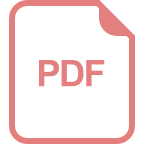
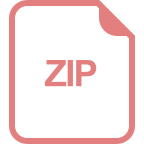
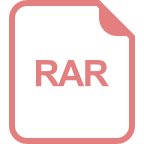
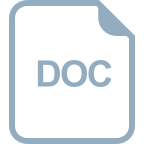
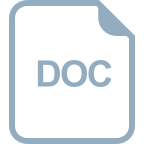
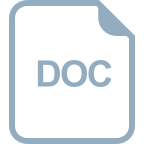
