Java代码In the rest of this question, use the readLine and readPosInt methods every time your program needs to read a string or an integer from the user. In the empty main method of the CLI class, create a single Library object with the name "UIC Library". The main method of the CLI class must then print a menu that allows the user of your system to do six different actions that involve the library object, and your program must then read an integer from the user that indicates which action must be performed by the program (see below for the details about each action). Use the readPosInt method to print the menu (give the string for the menu as the argument of readPosInt) and to read the integer typed by the user. For example, the menu should look like this: Type an action (total:1 add:2 get:3 more:4 less:5 quit:6): The user then types an integer between 1 and 6 to select the action. For example (where 3 is an input from the user): Type an action (total:1 add:2 get:3 more:4 less:5 quit:6): 3 and your program then performs the selected action.
时间: 2023-06-15 19:04:29 浏览: 107
Here is an example implementation of the CLI class:
```java
import java.util.Scanner;
public class CLI {
public static void main(String[] args) {
Library library = new Library("UIC Library");
Scanner scanner = new Scanner(System.in);
while (true) {
int choice = readPosInt("Type an action (total:1 add:2 get:3 more:4 less:5 quit:6): ");
switch (choice) {
case 1:
System.out.println("Total number of books in the library: " + library.getTotalBooks());
break;
case 2:
String title = readLine("Enter the title of the book to add: ");
String author = readLine("Enter the author of the book to add: ");
Book book = new Book(title, author);
library.addBook(book);
System.out.println("Book added successfully.");
break;
case 3:
String searchTitle = readLine("Enter the title of the book to search: ");
Book foundBook = library.getBook(searchTitle);
if (foundBook != null) {
System.out.println(foundBook.toString());
} else {
System.out.println("Book not found.");
}
break;
case 4:
int moreBooks = readPosInt("Enter the number of books to add to the library: ");
library.addBooks(moreBooks);
System.out.println("Books added successfully.");
break;
case 5:
int lessBooks = readPosInt("Enter the number of books to remove from the library: ");
boolean success = library.removeBooks(lessBooks);
if (success) {
System.out.println("Books removed successfully.");
} else {
System.out.println("Cannot remove more books than available in the library.");
}
break;
case 6:
System.out.println("Exiting the program...");
scanner.close();
System.exit(0);
default:
System.out.println("Invalid input, please try again.");
break;
}
}
}
private static String readLine(String prompt) {
System.out.print(prompt);
Scanner scanner = new Scanner(System.in);
return scanner.nextLine();
}
private static int readPosInt(String prompt) {
int i = 0;
boolean validInput = false;
Scanner scanner = new Scanner(System.in);
while (!validInput) {
System.out.print(prompt);
try {
i = Integer.parseInt(scanner.nextLine());
if (i > 0) {
validInput = true;
} else {
System.out.println("Please enter a positive integer.");
}
} catch (NumberFormatException e) {
System.out.println("Please enter a valid integer.");
}
}
return i;
}
}
```
This implementation creates a Library object, and then enters a loop where it prints a menu and reads an integer input from the user. Depending on the user's choice, it performs one of six actions on the Library object. The readLine and readPosInt methods are implemented as private static methods for convenience.
阅读全文
相关推荐



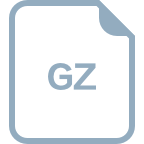





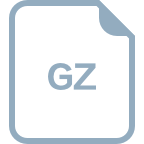
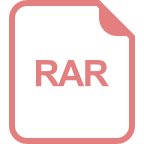






