下面这段代码要实现对学生对象按照成绩降序排序。 仔细阅读代码,要求实现编程实现输出运算符“<<”和小于“<”运算符,使本程序能完成预定的排序功能。 裁判测试程序样例: #include <iostream> #include <string> #include <list> using namespace std; class Student { string name; char sex; int score; string grade; public: Student(string name, char sex, int score, string grade); friend ostream &operator<< (ostream& os, Student st) ; friend bool operator<(Student &st1, Student &st2); }; //你提交的代码将被嵌入到这里 Student::Student(string name, char sex, int score, string grade) { this->name = name; this->sex = sex; this->score = score; this->grade = grade; } int main() { list<Student> st; string name, grade; char sex; int score; for(int i = 0; i < 5; i++) { cin>>name; cin>>sex; cin>>score; cin>>grade; st.push_back(Student(name, sex, score, grade)); } st.sort(); list<Student>::iterator p = st.begin(); while(p != st.end()) { cout<<*p; p++; } return 0; } 输入样例: 在这里给出一组输入。例如: Bill M 86 JK1501 David M 98 JK1502 Mary F 78 JK1503 Adam M 83 JK1504 Rose F 96 JK1505 输出样例: 在这里给出相应的输出。例如: David M 98 JK1502 Rose F 96 JK1505 Bill M 86 JK1501 Adam M 83 JK1504 Mary F 78 JK1503
时间: 2024-03-15 21:45:59 浏览: 132
以下是实现了输出运算符“<<”和小于“<”运算符的代码:
```c++
#include <iostream>
#include <string>
#include <list>
using namespace std;
class Student {
string name;
char sex;
int score;
string grade;
public:
Student(string name, char sex, int score, string grade);
friend ostream &operator<< (ostream& os, Student st);
friend bool operator<(Student &st1, Student &st2);
};
Student::Student(string name, char sex, int score, string grade) {
this->name = name;
this->sex = sex;
this->score = score;
this->grade = grade;
}
ostream &operator<< (ostream& os, Student st) {
os << st.name << " " << st.sex << " " << st.score << " " << st.grade << endl;
return os;
}
bool operator<(Student &st1, Student &st2) {
return st1.score > st2.score;
}
int main() {
list<Student> st;
string name, grade;
char sex;
int score;
for(int i = 0; i < 5; i++) {
cin >> name;
cin >> sex;
cin >> score;
cin >> grade;
st.push_back(Student(name, sex, score, grade));
}
st.sort();
list<Student>::iterator p = st.begin();
while(p != st.end()) {
cout << *p;
p++;
}
return 0;
}
```
在上面的代码中,我们重载了输出运算符“<<”和小于“<”运算符,分别用来输出学生信息和比较学生成绩。在主函数中,我们通过list容器存储学生对象,并使用sort函数来进行排序,最后输出排序后的结果。
阅读全文
相关推荐
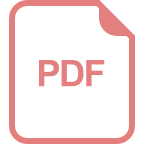
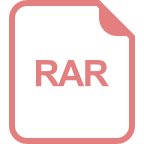
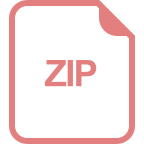
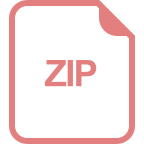
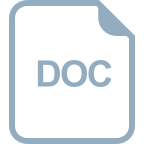
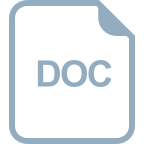
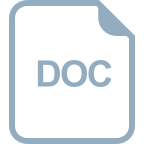
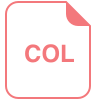
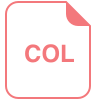
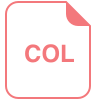
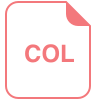
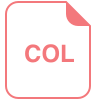
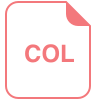
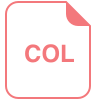
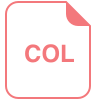
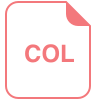