pycharm实现gui文档保护
时间: 2023-07-18 12:09:01 浏览: 142
您可以使用PyQt或Tkinter等Python GUI框架来实现GUI文档保护。以下是使用PyQt实现GUI文档保护的基本步骤:
1. 安装PyQt库。您可以使用pip命令进行安装。
2. 导入必要的PyQt模块。例如:
```python
from PyQt5.QtWidgets import QApplication, QMainWindow, QTextEdit, QAction, QFileDialog
from PyQt5.QtGui import QIcon
from PyQt5.QtCore import QFile
```
3. 创建主窗口,并添加菜单栏和工具栏。例如:
```python
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
# 创建文本编辑框
self.textEdit = QTextEdit()
self.setCentralWidget(self.textEdit)
# 添加菜单栏
menubar = self.menuBar()
fileMenu = menubar.addMenu('文件')
protectAction = QAction('文档保护', self)
protectAction.triggered.connect(self.protectDoc)
fileMenu.addAction(protectAction)
# 添加工具栏
toolbar = self.addToolBar('保护')
toolbar.addAction(protectAction)
self.show()
def protectDoc(self):
# 在此处添加文档保护的代码
pass
```
4. 实现文档保护的功能。您可以使用QFileDialog对话框来选择要保护的文件,使用QFile类来读取文件内容并进行加密,然后再将加密后的内容写回到原文件中。例如:
```python
def protectDoc(self):
fileName, _ = QFileDialog.getOpenFileName(self, '选择要保护的文件', '.', '文本文件 (*.txt)')
if fileName:
# 读取文件内容并进行加密
with open(fileName, 'r') as f:
content = f.read()
encryptedContent = encrypt(content)
# 将加密后的内容写回到原文件中
with open(fileName, 'w') as f:
f.write(encryptedContent)
QMessageBox.information(self, '提示', '文档已成功保护!')
```
其中,encrypt函数是您自己实现的加密函数。
希望这些信息能够帮助您实现GUI文档保护。
阅读全文
相关推荐
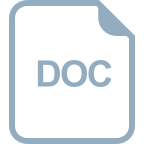
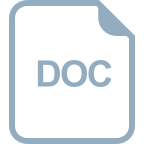
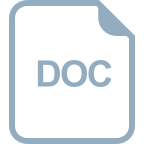

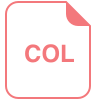
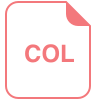
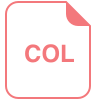
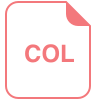
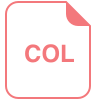
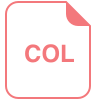








