python通过读取txt文件中的数字(txt文件为每行一个数字),绘制数字三角形的图(每个数字作为节点,每个节点用线段链接),并使用Q-learning算法寻找使最佳路径使该路径上的数字加和最大并绘制最佳路径图
时间: 2024-05-02 11:19:27 浏览: 8
这是一个非常复杂的问题,需要涉及到很多知识点,比如Python文件读取、图形绘制、Q-learning算法等,需要有一定的编程经验和数学基础才能完成。
以下是一些可能有用的代码片段:
1. 读取txt文件中的数字
```python
with open('filename.txt', 'r') as f:
nums = [int(line.strip()) for line in f.readlines()]
```
2. 绘制数字三角形的图
```python
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
for i in range(n):
for j in range(i+1):
x, y = j, -i
num = nums[i*(i+1)//2+j]
ax.text(x, y, num)
if i < n-1:
ax.plot([x, x+1], [y, y-1], color='gray')
ax.plot([x, x-1], [y, y-1], color='gray')
ax.set_xlim(-1, n)
ax.set_ylim(-n, 1)
plt.show()
```
3. Q-learning算法
```python
import numpy as np
q_table = np.zeros((n, n))
alpha = 0.1
gamma = 0.9
epsilon = 0.1
for episode in range(100):
state = (0, 0)
while state[0] < n-1:
if np.random.rand() < epsilon:
action = np.random.choice([0, 1])
else:
action = np.argmax(q_table[state])
if action == 0:
next_state = (state[0]+1, state[1])
else:
next_state = (state[0]+1, state[1]+1)
reward = nums[next_state[0]*(next_state[0]+1)//2+next_state[1]]
q_table[state][action] += alpha * (reward + gamma * np.max(q_table[next_state]) - q_table[state][action])
state = next_state
```
4. 绘制最佳路径图
```python
fig, ax = plt.subplots()
for i in range(n):
for j in range(i+1):
x, y = j, -i
num = nums[i*(i+1)//2+j]
ax.text(x, y, num)
if i < n-1:
ax.plot([x, x+1], [y, y-1], color='gray')
ax.plot([x, x-1], [y, y-1], color='gray')
state = (0, 0)
total_reward = 0
while state[0] < n-1:
action = np.argmax(q_table[state])
next_state = (state[0]+1, state[1]+action)
reward = nums[next_state[0]*(next_state[0]+1)//2+next_state[1]]
total_reward += reward
ax.plot([state[1], next_state[1]], [-state[0], -next_state[0]], color='red')
state = next_state
ax.set_xlim(-1, n)
ax.set_ylim(-n, 1)
plt.show()
```
这些代码片段只是一个大致的思路,实现起来还需要做很多细节上的处理,比如处理边界条件、调整图形大小和字体大小等。同时,Q-learning算法也需要进行调参,比如调整学习率、折扣因子和探索率等。
相关推荐
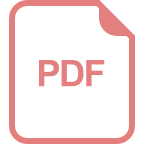
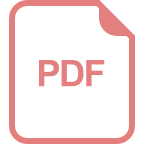














