elementui table二次封装
时间: 2023-07-25 19:20:31 浏览: 150
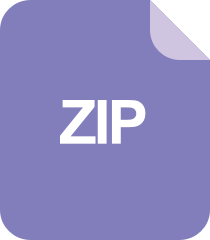
ElementUI Table二次封装
ElementUI 的 table 组件提供了非常丰富的功能和接口,但是在实际项目中,我们经常需要对其进行二次封装,以满足自己的业务需求。下面是一个简单的 ElementUI table 的二次封装示例:
```vue
<template>
<el-table
:data="tableData"
:height="height"
:max-height="maxHeight"
:stripe="stripe"
:border="border"
:fit="fit"
:show-header="showHeader"
:highlight-current-row="highlightCurrentRow"
:row-class-name="rowClassName"
:row-style="rowStyle"
:cell-class-name="cellClassName"
:cell-style="cellStyle"
:header-row-class-name="headerRowClassName"
:header-row-style="headerRowStyle"
:header-cell-class-name="headerCellClassName"
:header-cell-style="headerCellStyle"
:row-key="rowKey"
@select="handleSelect"
@select-all="handleSelectAll"
@selection-change="handleSelectionChange"
@cell-mouse-enter="handleCellMouseEnter"
@cell-mouse-leave="handleCellMouseLeave"
@cell-click="handleCellClick"
@cell-dblclick="handleCellDblClick"
@row-click="handleRowClick"
@row-contextmenu="handleRowContextMenu"
@row-dblclick="handleRowDblClick"
@header-click="handleHeaderClick"
@header-contextmenu="handleHeaderContextMenu"
@sort-change="handleSortChange"
@filter-change="handleFilterChange"
>
<slot></slot>
</el-table>
</template>
<script>
export default {
name: "MyTable",
props: {
tableData: {
type: Array,
default: () => []
},
height: {
type: [Number, String],
default: ""
},
maxHeight: {
type: [Number, String],
default: ""
},
stripe: {
type: Boolean,
default: true
},
border: {
type: Boolean,
default: true
},
fit: {
type: Boolean,
default: true
},
showHeader: {
type: Boolean,
default: true
},
highlightCurrentRow: {
type: Boolean,
default: true
},
rowClassName: {
type: Function,
default: () => undefined
},
rowStyle: {
type: Function,
default: () => undefined
},
cellClassName: {
type: Function,
default: () => undefined
},
cellStyle: {
type: Function,
default: () => undefined
},
headerRowClassName: {
type: Function,
default: () => undefined
},
headerRowStyle: {
type: Function,
default: () => undefined
},
headerCellClassName: {
type: Function,
default: () => undefined
},
headerCellStyle: {
type: Function,
default: () => undefined
},
rowKey: {
type: [String, Function],
default: ""
}
},
methods: {
handleSelect(selection, row) {
this.$emit("select", selection, row);
},
handleSelectAll(selection) {
this.$emit("select-all", selection);
},
handleSelectionChange(selection) {
this.$emit("selection-change", selection);
},
handleCellMouseEnter(row, column, cell, event) {
this.$emit("cell-mouse-enter", row, column, cell, event);
},
handleCellMouseLeave(row, column, cell, event) {
this.$emit("cell-mouse-leave", row, column, cell, event);
},
handleCellClick(row, column, cell, event) {
this.$emit("cell-click", row, column, cell, event);
},
handleCellDblClick(row, column, cell, event) {
this.$emit("cell-dblclick", row, column, cell, event);
},
handleRowClick(row, event, column) {
this.$emit("row-click", row, event, column);
},
handleRowContextMenu(row, event) {
this.$emit("row-contextmenu", row, event);
},
handleRowDblClick(row, event) {
this.$emit("row-dblclick", row, event);
},
handleHeaderClick(column, event) {
this.$emit("header-click", column, event);
},
handleHeaderContextMenu(column, event) {
this.$emit("header-contextmenu", column, event);
},
handleSortChange({ column, prop, order }) {
this.$emit("sort-change", { column, prop, order });
},
handleFilterChange(filters) {
this.$emit("filter-change", filters);
}
}
};
</script>
```
这里我们定义了一个名为 `MyTable` 的组件,并将其 props 与 ElementUI table 组件的 props 一一对应。在组件的模板中,我们使用了 `<slot></slot>`,表示组件的插槽,这样可以让用户在使用组件时自定义表格的列和内容。在组件的方法中,我们将 ElementUI table 的事件通过 `$emit` 的方式向上传递,这样可以使得组件的使用更加灵活。
使用这个组件时,我们只需要在父组件中引入 `MyTable` 组件,并将需要的 props 和插槽传入即可:
```vue
<template>
<my-table
:table-data="tableData"
:stripe="false"
:highlight-current-row="false"
@row-click="handleRowClick"
>
<el-table-column label="姓名" prop="name"></el-table-column>
<el-table-column label="年龄" prop="age"></el-table-column>
<el-table-column label="地址" prop="address"></el-table-column>
</my-table>
</template>
<script>
import MyTable from "@/components/MyTable";
export default {
name: "MyPage",
components: {
MyTable
},
data() {
return {
tableData: [
{
name: "张三",
age: 18,
address: "北京市"
},
{
name: "李四",
age: 20,
address: "上海市"
},
{
name: "王五",
age: 22,
address: "广州市"
}
]
};
},
methods: {
handleRowClick(row) {
console.log(row);
}
}
};
</script>
```
这样,我们就完成了一个简单的 ElementUI table 的二次封装,并且可以在父组件中灵活使用。
阅读全文
相关推荐
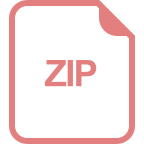
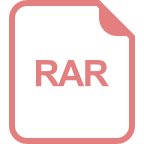
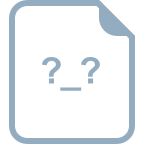
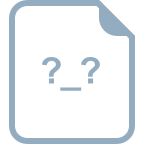
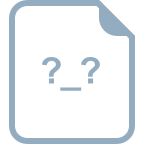
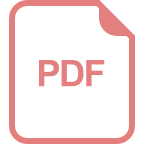
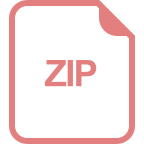
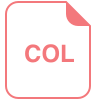





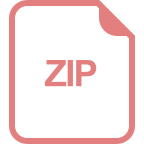
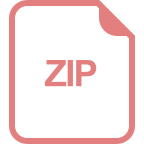
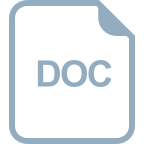

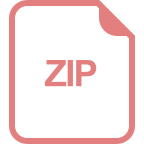