droplist=[] for i in range(len(df)): if (df['应发库'][i]!="sz"and df['应发库'][i]!="cs"and df['应发库'][i]!="sy"and df['应发库'][i]!="sh"and df['应发库'][i]!="cd"and df['应发库'][i]!="xa"and df['应发库'][i]!="km"and df['应发库'][i]!="jn"and df['应发库'][i]!="bj"): droplist.append(i) df2=df1.drop(labels=droplist,axis=0)这段代码报KeyError: '[357143] not found in axis'的原因
时间: 2023-08-30 20:06:12 浏览: 243
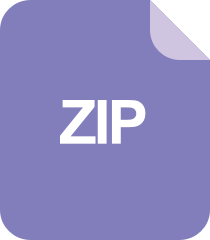
droplist:模拟选择
这个错误通常是由于尝试删除不存在于数据框中的行所引起的。具体来说,代码中 droplist 列表中的索引可能包含了数据框 df1 中不存在的行索引,导致在执行 `drop()` 方法时出现 KeyError 错误。
你可以添加以下代码来检查 droplist 列表中的索引是否超出了 df1 的行数范围:
```python
if max(droplist) >= len(df1):
print("索引超出范围")
```
如果输出结果为 “索引超出范围”,则需要检查代码中的索引是否正确。
阅读全文
相关推荐
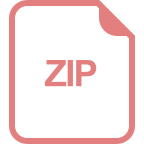
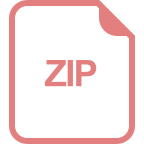

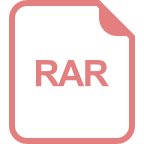
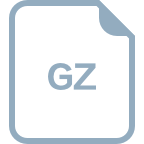
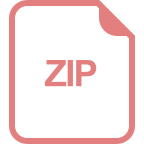
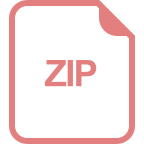
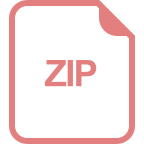
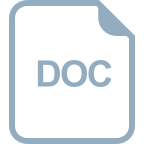
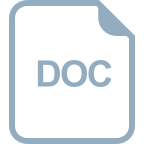
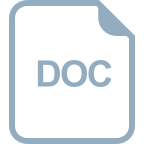





