Java 编写html解码器
时间: 2023-07-11 15:54:25 浏览: 74
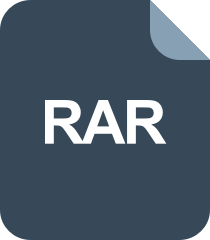
java的html编辑器

在Java中,可以使用`java.net.URLDecoder`类来解码HTML编码,使用`java.util.Base64`类来解码HTML5编码。
以下是一个简单的HTML解码器实现:
```java
import java.net.URLDecoder;
import java.util.Base64;
public class HtmlDecoder {
public static void main(String[] args) {
String html = "Hello%20World!<br />SGVsbG8gV29ybGQh";
String decodedHtml = decodeHtml(html);
System.out.println(decodedHtml);
}
public static String decodeHtml(String html) {
String decodedHtml = html;
decodedHtml = decodeHtml5(decodedHtml);
decodedHtml = decodeHtmlEntities(decodedHtml);
return decodedHtml;
}
public static String decodeHtml5(String html) {
String decodedHtml = html;
while (decodedHtml.contains("<") && decodedHtml.contains(">")) {
int startIndex = decodedHtml.indexOf("<");
int endIndex = decodedHtml.indexOf(">", startIndex);
if (startIndex >= 0 && endIndex >= 0) {
String encodedString = decodedHtml.substring(startIndex + 4, endIndex);
String decodedString = new String(Base64.getDecoder().decode(encodedString));
decodedHtml = decodedHtml.substring(0, startIndex) + decodedString + decodedHtml.substring(endIndex + 4);
}
}
return decodedHtml;
}
public static String decodeHtmlEntities(String html) {
String decodedHtml = html;
try {
decodedHtml = URLDecoder.decode(decodedHtml, "UTF-8");
} catch (Exception e) {
e.printStackTrace();
}
return decodedHtml;
}
}
```
在`decodeHtml()`方法中,先调用`decodeHtml5()`方法对HTML5编码进行解码,然后调用`decodeHtmlEntities()`方法对HTML实体进行解码。
在`decodeHtml5()`方法中,使用`Base64.getDecoder().decode()`方法对HTML5编码进行解码,然后使用`String.substring()`方法将解码后的字符串替换为原字符串中的HTML5编码。
在`decodeHtmlEntities()`方法中,使用`URLDecoder.decode()`方法对HTML实体进行解码。
需要注意的是,在解码HTML5编码时,需要先判断字符串中是否包含"<"和">",否则会出现解码错误的情况。
阅读全文
相关推荐
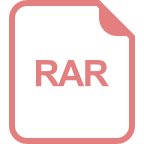
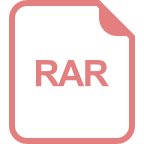
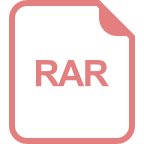
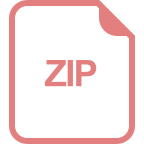
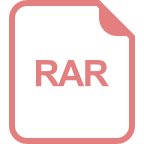
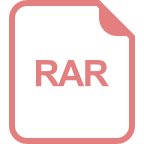
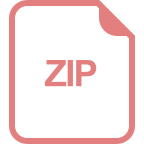
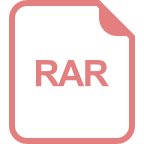
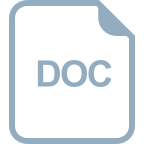
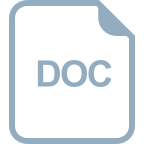
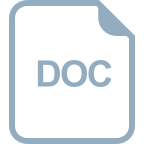
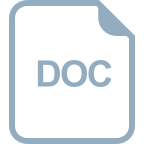
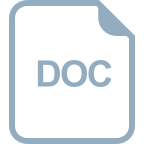
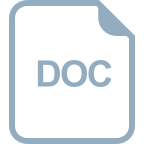
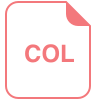
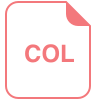