python平凡英雄电影影评情感分析代码
时间: 2023-07-22 22:41:39 浏览: 48
以下是一个基于Python的简单电影影评情感分析代码,使用了NLTK库和情感词典SentiWordNet:
```python
import nltk
from nltk.corpus import sentiwordnet as swn
# 下载NLTK的停用词和词性标注器
nltk.download('stopwords')
nltk.download('averaged_perceptron_tagger')
# 定义函数,输入为一个句子,输出为情感得分(介于-1和1之间)
def sentiment_score(sentence):
# 定义情感得分变量
pos_score = 0
neg_score = 0
# 分词和词性标注
tokens = nltk.word_tokenize(sentence.lower())
tagged_tokens = nltk.pos_tag(tokens)
# 对于每个词,查找其对应的情感得分
for word, tag in tagged_tokens:
# 过滤掉停用词和标点符号
if word not in nltk.corpus.stopwords.words('english') and word.isalpha():
# 根据词性和词义查找情感得分
synsets = list(swn.senti_synsets(word))
if synsets:
# 取第一个词义的情感得分
synset = synsets[0]
pos_score += synset.pos_score()
neg_score += synset.neg_score()
# 计算情感得分
if pos_score == 0 and neg_score == 0:
return 0
else:
return (pos_score - neg_score) / (pos_score + neg_score)
# 读取电影影评数据
with open('reviews.txt', 'r', encoding='utf-8') as f:
reviews = f.readlines()
# 计算每个影评的情感得分
scores = []
for review in reviews:
score = sentiment_score(review)
scores.append(score)
# 计算平均得分和正面评价比例
avg_score = sum(scores) / len(scores)
pos_ratio = len([score for score in scores if score > 0]) / len(scores)
# 输出结果
print('平均得分:', avg_score)
print('正面评价比例:', pos_ratio)
```
需要注意的是,这只是一个简单的示例代码,情感分析的结果可能会受到词义歧义、否定词、情感强度等因素的影响,因此对于更准确的情感分析,需要使用更复杂的算法和技术。
相关推荐
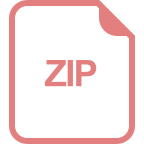
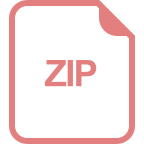














