编程实现字符串连接函数strcat()的功能。c语言
时间: 2024-11-12 11:40:57 浏览: 43
在 C 语言中,`strcat()` 函数用于将一个字符串追加到另一个字符串的末尾。它把第二个字符串的内容直接复制到第一个字符串的内存空间中,所以第一个字符串需要有足够的空间容纳附加的字符串。以下是 `strcat()` 的基本使用方法和一个简单的实现示例(尽管在实际项目中通常会使用库函数,这里仅作教学目的):
```c
#include <stdio.h>
#include <string.h> // 引入strcat() 函数声明
// 使用指针实现的简单 strcat()
char* my_strcat(char* dest, const char* src) {
// 检查目标字符串是否已到达其最大容量
if (!dest || !src) {
printf("Error: Invalid arguments to strcat.\n");
return NULL;
}
// 指向dest的最后一个字符
char* dest_end = dest + strlen(dest) - 1;
// 将src逐个字符复制到dest,直到遇到'\0'或src结束
while (*src) {
*dest_end++ = *src++;
}
// 在src末尾添加'\0',表示字符串结束
*dest_end = '\0';
return dest;
}
int main() {
char destination[50] = "Hello, ";
char source[] = "World!";
strcat(destination, source); // 使用系统函数或my_strcat()
printf("Destination string after strcat: %s\n", destination);
return 0;
}
```
当运行这段代码时,最终的`destination`字符串将会是"Hello, World!"。
阅读全文
相关推荐




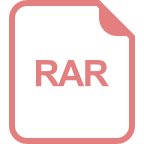
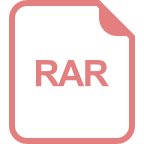










