如何在简单的工厂模式中编写一个计算不同几何形状(如矩形、三角形、圆形等)面积的示例代码?
时间: 2024-12-15 16:13:59 浏览: 13
在简单的工厂模式中,我们可以创建一个工厂类,该类包含一个或多个静态方法,每个方法对应一种几何形状,返回对应的面积计算器对象。下面是一个Java示例代码:
```java
// 定义一个抽象的几何形状接口
interface Shape {
double getArea();
}
// 实现具体的几何形状类
class Rectangle implements Shape {
private double width, height;
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
@Override
public double getArea() {
return width * height;
}
}
class Triangle implements Shape {
private double base, height;
public Triangle(double base, double height) {
this.base = base;
this.height = height;
}
@Override
public double getArea() {
return 0.5 * base * height;
}
}
class Circle implements Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
public double getArea() {
return Math.PI * radius * radius;
}
}
// 创建工厂类
class GeometryFactory {
// 使用工厂方法创建特定类型的面积计算器
public static Shape createShape(String shapeType, double... dimensions) {
switch (shapeType) {
case "Rectangle":
return new Rectangle(dimensions[0], dimensions[1]);
case "Triangle":
return new Triangle(dimensions[0], dimensions[1]); // 假设三角形提供两边长
case "Circle":
return new Circle(dimensions[0]); // 假设圆的半径是一个参数
default:
throw new IllegalArgumentException("Invalid shape type");
}
}
}
// 测试代码
public class Main {
public static void main(String[] args) {
Shape rectangle = GeometryFactory.createShape("Rectangle", 5, 6); // 矩形
System.out.println("Rectangle area: " + rectangle.getArea());
Shape triangle = GeometryFactory.createShape("Triangle", 3, 4); // 三角形
System.out.println("Triangle area: " + triangle.getArea());
Shape circle = GeometryFactory.createShape("Circle", 3); // 圆形
System.out.println("Circle area: " + circle.getArea());
}
}
```
在这个例子中,`GeometryFactory`类负责创建各种形状的对象,客户端通过传入形状类型和必要的参数来获取相应的面积计算器。
阅读全文
相关推荐
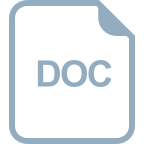
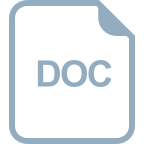
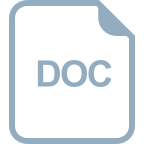
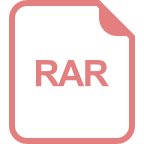
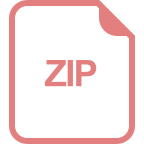
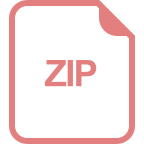
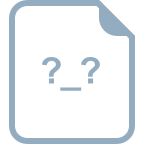
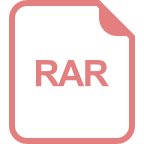
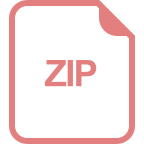
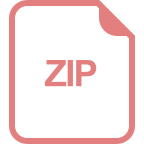
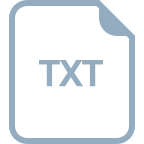
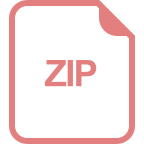
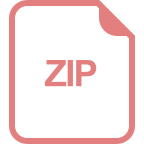
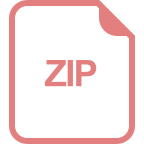
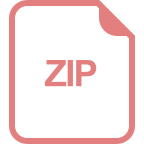
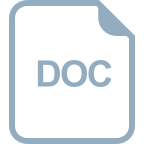
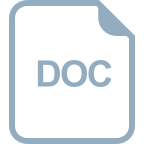
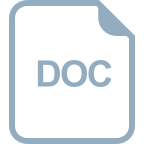
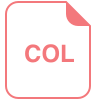