java 给定word文件路径转pdf后,把pdf上传到文件服务器
时间: 2024-10-25 12:02:09 浏览: 41
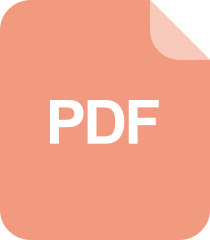
java生成word文件并下载.pdf
在Java中,将Word文档转换成PDF并上传到文件服务器通常需要几个步骤:
1. **安装库**:
- 使用Apache POI处理Word文档(`.docx`),它提供读取和操作Word文档的功能。
- 使用iText库或Flying Saucer等工具将Word转换为PDF。
- 使用Java的HttpURLConnection、HttpClient或第三方库如Spring框架的RestTemplate来进行文件上传。
2. **转换步骤**:
```java
// 导入所需库
import com.itextpdf.text.Document;
import com.itextpdf.text.pdf.PdfWriter;
try {
// 读取Word文件
InputStream wordStream = new FileInputStream(wordFilePath);
WordToPdfConverter converter = new WordToPdfConverter(wordStream);
// 创建一个新的PDF
Document document = new Document();
PdfWriter.getInstance(document, new FileOutputStream(pdfFilePath));
document.open();
// 转换并写入PDF
converter.convertToPdf(document);
// 关闭资源
document.close();
wordStream.close();
} catch (Exception e) {
e.printStackTrace();
}
```
3. **文件上传**:
```java
// 假设你已经有了一个URL指向文件服务器
URL uploadUrl = new URL(serverUploadPath);
HttpURLConnection connection = (HttpURLConnection) uploadUrl.openConnection();
connection.setRequestMethod("PUT");
connection.setRequestProperty("Content-Type", "application/pdf");
File pdfFile = new File(pdfFilePath);
Files.copy(pdfFile.toPath(), connection.getOutputStream());
int responseCode = connection.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
System.out.println("PDF uploaded successfully.");
} else {
System.err.println("Failed to upload PDF with error code: " + responseCode);
}
connection.disconnect();
```
注意:这个例子假设你已经实现了`WordToPdfConverter`,实际项目中可能需要查找现成的库来完成转换,如Aspose.Words for Java。同时,为了简化代码,一些错误处理和异常捕获在这里省略了。
阅读全文
相关推荐
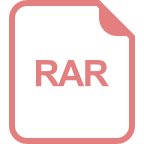
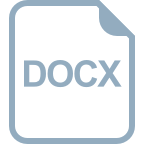
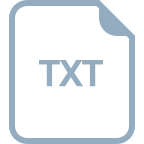
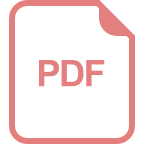
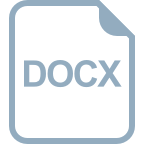
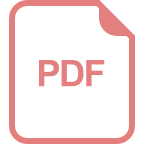
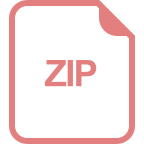
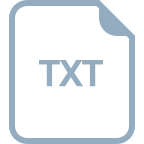
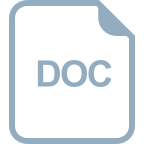
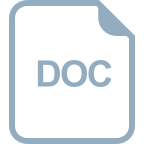
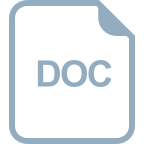
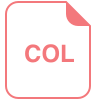
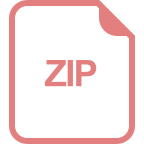
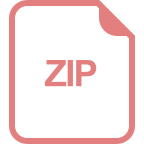