建立一个c++顺序表,实现输入通讯公司名字,查询到使用该通讯公司号码的全部同学信息
时间: 2024-09-25 09:04:57 浏览: 35
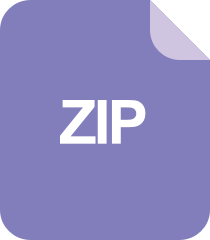
C++实现蓝牙bluetooth通讯功能
在C++中,建立一个顺序表(如动态数组或简单的结构体链表)来存储同学信息和通讯公司的对应关系,可以按照以下步骤进行:
首先,定义一个包含姓名、电话和通讯公司名称的数据结构,例如`StudentInfo`:
```cpp
struct StudentInfo {
string name;
string phone_number;
string telecom_company;
};
```
接着,创建一个`StudentList`类,它有一个动态大小的数组或者指针来存储`StudentInfo`对象,以及用于添加和查找学生的方法:
```cpp
class StudentList {
private:
std::vector<StudentInfo> students; // 使用动态数组实现顺序表
public:
void addStudent(const string& name, const string& phone, const string& company) {
StudentInfo new_student = {name, phone, company};
students.push_back(new_student);
}
// 通过通讯公司名称查找所有相关学生
vector<StudentInfo> searchByCompany(const string& company) {
vector<StudentInfo> matching_students;
for (const auto& student : students) {
if (student.telecom_company == company) {
matching_students.push_back(student);
}
}
return matching_students;
}
};
```
现在你可以创建一个`StudentList`实例,并使用上述方法添加学生和查询特定通讯公司的学生信息:
```cpp
int main() {
StudentList school;
// 添加学生
school.addStudent("张三", "13800138000", "电信");
school.addStudent("李四", "13900139000", "电信");
school.addStudent("王五", "13600136000", "移动");
// 查询电信公司的学生
vector<StudentInfo> telecom_students = school.searchByCompany("电信");
for (const auto& student : telecom_students) {
cout << "Name: " << student.name << ", Phone: " << student.phone_number << endl;
}
return 0;
}
```
阅读全文
相关推荐
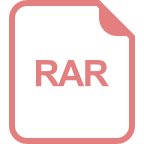
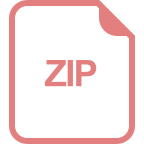
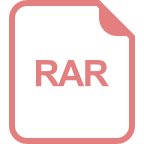
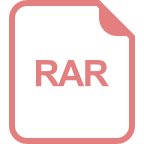
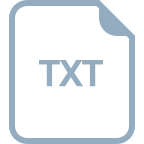
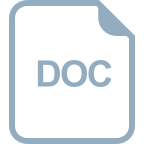
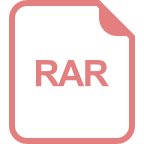
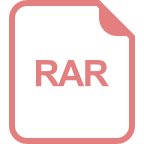
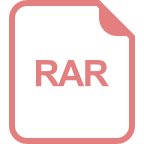
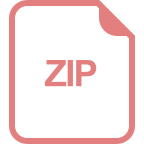
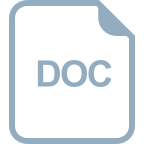
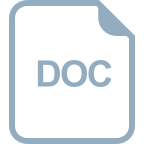
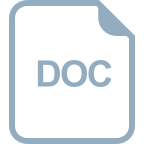
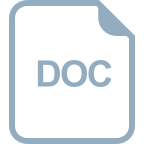
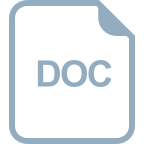
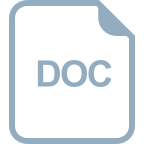