如何用c语言编写斐波那契数列
时间: 2024-10-06 14:00:56 浏览: 88
在C语言中编写斐波那契数列,你可以通过循环或者递归的方式来实现。以下是两种常见的方法:
**1. 循环法(迭代法)**:
```c
#include <stdio.h>
void fibonacci(int n) {
int t1 = 0, t2 = 1, nextTerm;
printf("Fibonacci Series up to %d terms:\n", n);
for (int i = 1; i <= n; ++i) {
if(i == 1 || i == 2) {
nextTerm = i;
} else {
nextTerm = t1 + t2;
t1 = t2;
t2 = nextTerm;
}
printf("%d ", nextTerm);
}
}
int main() {
int numTerms;
printf("Enter the number of terms: ");
scanf("%d", &numTerms);
fibonacci(numTerms);
return 0;
}
```
**2. 递归法**:
```c
#include <stdio.h>
// 使用递归函数计算斐波那契数
int fibonacci(int n) {
if(n <= 1)
return n;
else
return(fibonacci(n-1) + fibonacci(n-2));
}
int main() {
int num;
printf("Enter a positive integer: ");
scanf("%d", &num);
if(num <= 0){
printf("Invalid input! Please enter a positive integer.\n");
} else {
printf("Fibonacci sequence for %d terms is: \n", num);
for(int i=0; i<num; ++i)
printf("%d, ", fibonacci(i));
}
return 0;
}
```
阅读全文
相关推荐
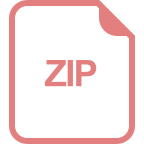
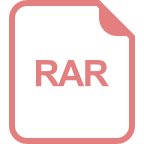
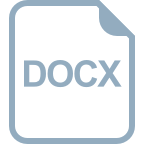



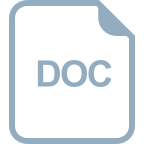











