如何判断一个字符串里面含有另一个字符串
时间: 2024-09-08 13:03:57 浏览: 82
判断一个字符串是否包含另一个字符串,通常有几种常见的方式:
1. **使用in关键字**:在Python中,你可以直接使用`in`关键字检查一个字符串是否在另一个字符串中出现,例如:
```python
s1 = "Hello World"
if "World" in s1:
print("包含")
else:
print("不包含")
```
2. **使用find()或index()方法**:这两种方法会查找子字符串在原字符串中的位置,如果找到则返回子字符串的起始索引,反之返回-1(find)或抛出异常(index)。如:
```python
s1 = "Hello World"
position = s1.find("World")
if position != -1:
print("包含")
```
请注意,`find()`方法对于大小写敏感,而`index()`方法会抛出异常。
3. **正则表达式**:如果你需要更复杂的匹配规则,可以使用Python的re模块来处理,比如`re.search()`函数。
无论哪种方法,都可以通过比较返回的结果或者不存在的位置信息来确定是否包含。
相关问题
如何判断一个字符串里面含有另一个字符串c++
在 C++ 中,你可以使用 `find` 函数或者 `contains` 集合函数来判断一个字符串是否包含另一个字符串。以下是两种常见方法:
1. 使用 `std::string` 类的 `find` 函数:
```cpp
#include <iostream>
#include <string>
bool containsString(std::string str1, std::string str2) {
if (str1.find(str2) != std::string::npos) {
return true;
}
else {
return false;
}
}
int main() {
std::string mainStr = "Hello, world!";
std::string substr = "world";
if (containsString(mainStr, substr)) {
std::cout << mainStr << " contains " << substr << std::endl;
} else {
std::cout << mainStr << " does not contain " << substr << std::endl;
}
return 0;
}
```
在这个例子中,`find` 如果找到 `str2` 就返回 `str2` 的起始位置,找不到则返回 `npos`。所以检查返回值是否等于 `npos` 可以确定是否包含。
2. 对于C++17及以上版本,可以使用标准库中的 `std::ranges::contains` 或者 Boost 库的 `boost::algorithm::contains`:
```cpp
#include <iostream>
#include <string>
#include <ranges> // For C++17 or later
bool containsString(std::string_view str1, std::string_view str2) {
return std::ranges::contains(str1, str2);
}
int main() {
std::string mainStr = "Hello, world!";
if (containsString(mainStr, "world")) {
// ...
}
return 0;
}
```
C#中怎么判断一个字符串里面是否含E
在C#中,你可以使用多种方法来判断一个字符串是否包含某个特定的字符或者子字符串。如果你要检查字符串中是否含有字符'E',可以使用`Contains`方法。这是一个简单而直接的例子:
```csharp
string testString = "Example String";
bool containsE = testString.Contains('E');
```
在这个例子中,`testString`是我们要检查的字符串,`Contains`方法用于检查它是否包含字符'E'。如果包含,则返回`true`;否则返回`false`。
你还可以使用`IndexOf`方法,它会返回子字符串在字符串中首次出现的位置的索引,如果没有找到则返回-1。
```csharp
string testString = "Example String";
int position = testString.IndexOf('E');
bool containsE = position != -1;
```
在这个例子中,`IndexOf`方法会返回'E'在`testString`中的位置索引。如果找到了'E',则`position`将不等于-1,于是`containsE`为`true`;如果没有找到,则`containsE`为`false`。
阅读全文
相关推荐
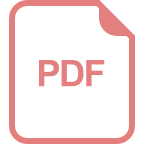
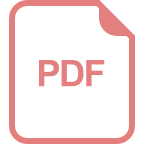
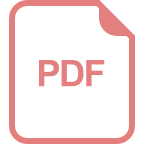













