aes加密解密python
时间: 2024-10-13 18:01:12 浏览: 13
AES(Advanced Encryption Standard),即高级加密标准,是一种常用的对称加密算法,安全性较高。在Python中,我们可以使用`cryptography`库来实现AES加密和解密。
首先,你需要安装这个库,可以使用pip命令:
```bash
pip install cryptography
```
然后,你可以按照以下步骤进行操作:
1. 导入必要的模块:
```python
from cryptography.hazmat.primitives.ciphers import Cipher, algorithms, modes
from cryptography.hazmat.backends import default_backend
```
2. 加密部分:
```python
def encrypt_AES(plaintext, key, iv):
backend = default_backend()
cipher = Cipher(algorithms.AES(key), modes.CBC(iv), backend=backend)
encryptor = cipher.encryptor()
ciphertext = encryptor.update(plaintext) + encryptor.finalize()
return ciphertext, iv
# 示例
key = b'sixteen byte key' # AES key应该是16、24或32字节
iv = b'some random IV' # 初始化向量(IV),用于保证每次加密的随机性
plaintext = b"Hello, AES!"
ciphertext, iv_used = encrypt_AES(plaintext, key, iv)
```
3. 解密部分:
```python
def decrypt_AES(ciphertext, key, iv):
backend = default_backend()
decipher = Cipher(algorithms.AES(key), modes.CBC(iv), backend=backend).decryptor()
plaintext = decipher.update(ciphertext) + decipher.finalize()
return plaintext
decrypted_text = decrypt_AES(ciphertext, key, iv_used)
```
注意:在实际应用中,IV需要妥善保管,并且每次加密时应使用新的随机IV。
阅读全文
相关推荐
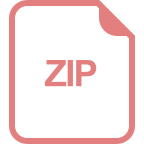
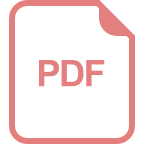




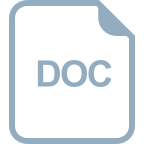


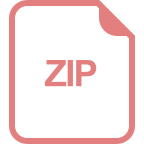
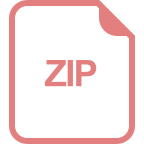
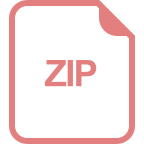