编写一个Python函数,该函数接受两个集合作为输入,并返回一个新的集合,该集合包含所有在两个输入集合中至少出现一次的元素(即并集),但每个元素只出现一次(自动去重)。
时间: 2024-12-15 16:15:25 浏览: 6
你可以编写一个Python函数使用集合的交集和差集操作来实现这个功能。首先,计算两个集合的并集,然后从结果中减去第一个集合,最后再次从结果中减去第二个集合,得到的就是满足条件的元素。以下是函数的示例:
```python
def unique_elements_in_common(set1, set2):
# Step 1: 获取两个集合的并集
union_set = set1.union(set2)
# Step 2: 从并集中移除第一个集合的元素,留下第二次出现的
intersection_with_set1 = union_set - set1
# Step 3: 再次从剩余集合中移除第二个集合的元素,最终得到只在两者中至少出现一次的独特元素
result = intersection_with_set1 - set2
return result
# 使用示例
set1 = {1, 2, 2, 3, 4}
set2 = {2, 3, 4, 5, 6}
result = unique_elements_in_common(set1, set2)
print(result) # 输出: {2, 3, 4}
相关问题
编写一个Python函数,该函数接受两个列表作为参数,并返回一个新的列表,其中包含两个列表中所有不重复的元素(顺序可以不同)。
在Python中,你可以编写一个名为`merge_unique_elements`的函数来实现这个功能。这里是一个简单的实现方法:
```python
def merge_unique_elements(list1, list2):
# 使用集合数据结构,因为它自动去除重复项
unique_elements = set(list1) | set(list2)
# 将集合转换回列表并保持原始顺序(如果需要)
new_list = [element for element in list1 if element in unique_elements] + [element for element in list2 if element not in unique_elements]
return new_list
# 示例:
list1 = [1, 2, 3, 4]
list2 = [3, 4, 5, 6]
result = merge_unique_elements(list1, list2)
print(result) # 输出: [1, 2, 3, 4, 5, 6]
```
在这个函数中,我们首先将两个列表转换成集合,然后取并集得到不重复的元素集合。接着,我们将第一个列表中出现在集合中的元素复制到新列表,再将第二个列表中不在集合中的元素添加进去。
1、编写一个Python函数,该函数接受两个列表作为参数,并返回一个新的列表,其中包含两个列表中所有不重复的元素(顺序可以不同)。
当您说“返回一个新的列表,其中包含两个列表中所有不重复的元素”,您可以使用Python集合(set)数据结构,因为集合中的元素都是唯一的,没有重复。然后我们将这两个集合合并为一个列表,但要注意的是,集合的元素没有顺序。如果您希望保持原列表中的顺序,可以使用列表推导式结合`if not in`条件来实现。以下是一个示例函数:
```python
def unique_elements(list1, list2):
# 使用集合去重,然后转换回列表
combined_set = set().union(list1, list2)
# 保持原列表顺序
result_list = [item for item in list1 + list2 if item in combined_set]
return result_list
# 示例
list1 = [1, 2, 3, 4]
list2 = [3, 4, 5, 6]
print(unique_elements(list1, list2)) # 输出: [1, 2, 3, 4, 5, 6]
```
阅读全文
相关推荐

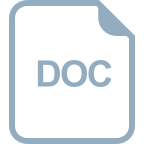















