学生成绩管理系统c语言devc++
时间: 2024-01-18 12:17:38 浏览: 148
学生成绩管理系统是一个用于管理学生信息和成绩的软件。在C语言中,可以使用链表来实现学生成绩管理系统。Dev-C++是一个常用的C语言集成开发环境(IDE),可以用于编写和运行C语言程序。
以下是一个使用链表实现学生成绩管理系统的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义学生结构体
typedef struct student {
int id;
char name[50];
float score;
struct student* next;
} Student;
// 添加学生信息
void addStudent(Student** head, int id, char name[], float score) {
Student* newStudent = (Student*)malloc(sizeof(Student));
newStudent->id = id;
strcpy(newStudent->name, name);
newStudent->score = score;
newStudent->next = NULL;
if (*head == NULL) {
*head = newStudent;
} else {
Student* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = newStudent;
}
}
// 显示学生信息
void displayStudents(Student* head) {
Student* current = head;
while (current != NULL) {
printf("ID: %d, Name: %s, Score: %.2f\n", current->id, current->name, current->score);
current = current->next;
}
}
int main() {
Student* head = NULL;
// 添加学生信息
addStudent(&head, 1, "Alice", 85.5);
addStudent(&head, 2, "Bob", 92.0);
addStudent(&head, 3, "Charlie", 78.5);
// 显示学生信息
displayStudents(head);
return 0;
}
```
这段代码实现了一个简单的学生成绩管理系统,可以添加学生信息并显示学生信息。你可以根据自己的需求进行扩展和修改。
阅读全文
相关推荐
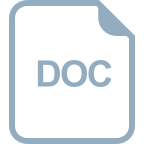
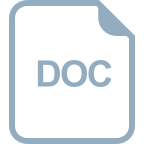
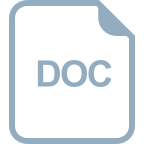
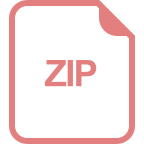
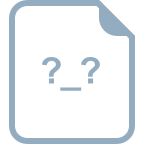
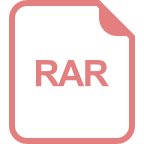
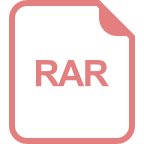
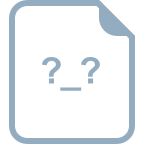
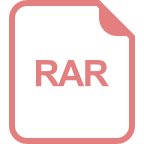
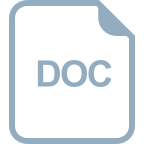
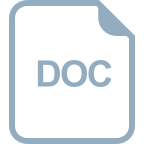
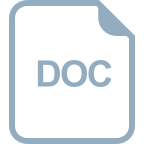
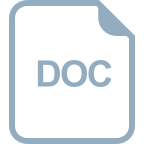

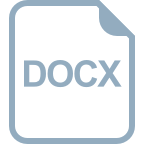



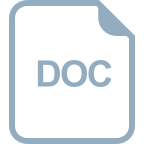