使用java通过M-ary乘方算法来实现模指数运算,并编写具体的java代码
时间: 2024-10-12 15:14:59 浏览: 52
M-ary乘方算法(也称为分治法或二分乘方)是一种用于高效计算大整数幂次运算的方法,特别适合于模运算,因为它的效率高于直接递归计算。在Java中,我们可以将大整数分解为较小的部分,然后逐位相乘,同时考虑到模运算的特性。
以下是使用M-ary乘方算法实现模指数运算的Java代码示例:
```java
import java.math.BigInteger;
public class M_aryExponentiation {
public static BigInteger modularExponentiation(BigInteger base, BigInteger exponent, BigInteger mod) {
if (mod.compareTo(BigInteger.ONE) <= 0 || base.compareTo(BigInteger.ZERO).equals(1)) {
throw new IllegalArgumentException("Invalid input for modular exponentiation");
}
// Base case: when exponent is zero or one
if (exponent.equals(BigInteger.ZERO)) {
return BigInteger.ONE;
} else if (exponent.mod(BigInteger.TWO).equals(BigInteger.ONE)) { // odd exponent case
return base.modPow(exponent.subtract(BigInteger.ONE), mod);
} else {
// Even exponent case: divide exponent by two and square the base
BigInteger half = exponent.divide(BigInteger.TWO);
BigInteger halfResult = modularExponentiation(base, half, mod);
return halfResult.multiply(halfResult).mod(mod);
}
}
public static void main(String[] args) {
BigInteger base = new BigInteger("13"); // replace with your desired base
BigInteger exponent = new BigInteger("123456789"); // replace with your desired exponent
BigInteger mod = new BigInteger("1000000007"); // replace with your desired modulus
BigInteger result = modularExponentiation(base, exponent, mod);
System.out.println("Modular Exponentiation Result: " + result);
}
}
```
在这个例子中,`modularExponentiation`函数接收三个参数:底数、指数和模数。它首先检查输入的有效性,然后通过递归地处理指数的奇偶性来进行计算。请注意,你需要替换`base`, `exponent`, 和 `mod`变量为你实际需要的值。
阅读全文
相关推荐
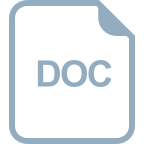
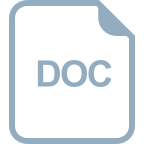
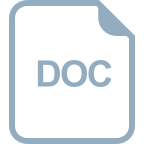
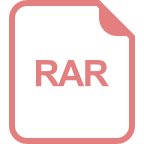
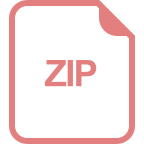
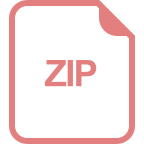
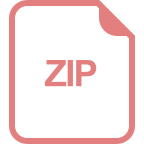
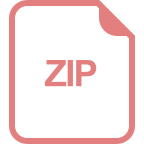
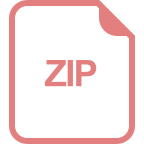
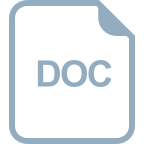
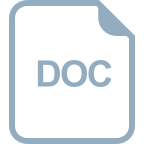
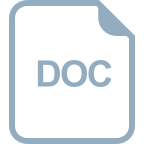
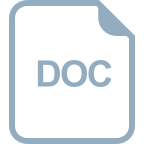



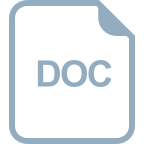
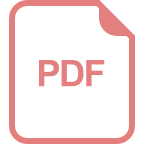
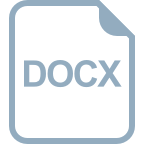