template <class T> class List { date1<T>* pre, * head, * p; int pos = 0; public: List() { this->head = new date1<T>; this->pre = head; } void push(T a) { if (pos <= 1) { p = new date1<T>; p->changedate(a); p->changenext(nullptr); pre->changenext(p); pre = p; pos++; } else if (pos > 1) { p = new date1<T>; p->changedate(a); p->changenext(nullptr); p->changelast(pre); pre->changenext(p); pre = p; pos++; } } void Delete() { date1<T>* a = pre->getlast(); delete[]pre; pre = a; pos--; } T sum() { T sum = 0; date1<T>* a = head->getnext(); while (a != nullptr) { sum += a->getdate(); a = a->getnext(); } return sum; } void order() { date1<T>* a = head->getnext(); date1<T>* b = a->getnext(); while (a->getnext() != nullptr) { while (b != nullptr) { if (a->getdate() <= b->getdate()) { T a1 = a->getdate(); a->changedate(b->getdate()); b->changedate(a1); } b = b->getnext(); } a = a->getnext(); } } void search(T a) { date1<T>* b=head; while (b!= nullptr) { if (b->date == a) { cout << "found it"; return; } b = b->getnext(); } cout << "no found"; } T average() { T sum1 =this->sum(); date1<T>* b = head; int i = 0; while (b != nullptr) { b = b->getnext; i++; } return sum/i; }找出average函数的不足之处并且优化他
时间: 2024-03-25 20:36:32 浏览: 54
average函数的不足之处是它没有考虑到空列表的情况,如果列表为空,它将会抛出除以零的异常。同时,它也没有使用浮点类型来存储平均值,如果对于整数类型的列表,可能会导致精度丢失。
为了优化average函数,我们可以先判断列表是否为空,如果为空则返回默认值,比如-1。另外,我们可以使用浮点类型来存储平均值,这样可以避免精度丢失的问题。
下面是修改后的average函数的代码:
```c++
double average() {
if (pos == 0) {
return -1; // 如果列表为空,返回-1
}
T sum1 = this->sum();
date1<T>* b = head;
int i = 0;
while (b != nullptr) {
b = b->getnext();
i++;
}
return static_cast<double>(sum1) / i; // 使用浮点类型存储平均值
}
```
阅读全文
相关推荐
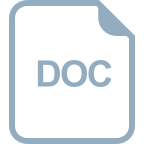
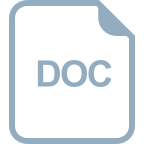
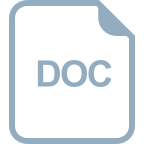
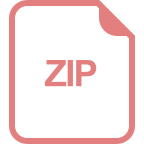
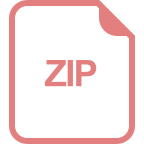
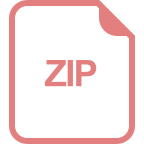
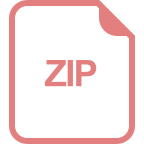
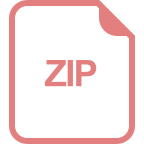
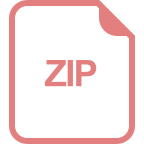
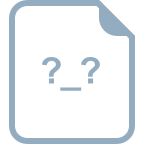
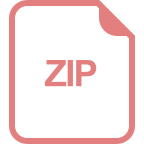
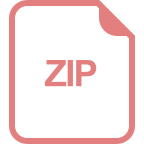
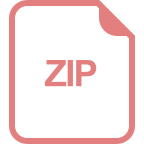
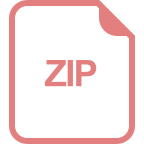
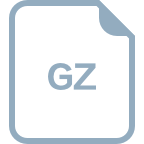
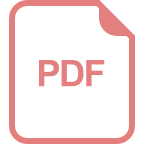
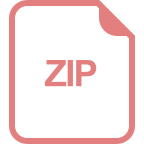
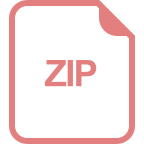
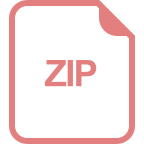