在Unity 中如何访问其他场景的变量
时间: 2024-02-21 07:02:09 浏览: 81
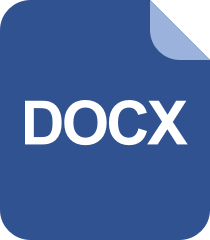
Unity3D脚本:如何调用更改其他脚本中的变量2
在Unity中,每个场景都是独立的,因此不能直接访问其他场景的变量。但是,你可以使用`SceneManager`类中的方法来加载其他场景并获取其变量的值。
具体步骤如下:
1. 在要访问的场景中,创建一个空的游戏对象,命名为`GameController`(或其他你喜欢的名字)。
2. 在`GameController`上添加一个脚本,定义你想要在其他场景中访问的变量,并将其声明为`public static`类型。例如:
```
public static int score = 0;
```
3. 在其他场景中的脚本中,使用以下代码加载`GameController`游戏对象,并访问其变量:
```
// 加载场景
SceneManager.LoadScene("MyScene");
// 获取GameController游戏对象
GameObject gameController = GameObject.Find("GameController");
// 获取变量的值
int myScore = gameController.GetComponent<GameController>().score;
```
这样,你就可以在其他场景中访问`GameController`中的变量了。注意,为了使此方法有效,必须保证在要访问的场景中已经加载了`GameController`游戏对象。如果没有加载,将会返回空引用错误。
阅读全文
相关推荐
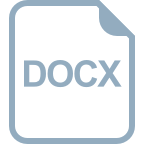
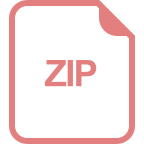















