java随机生成字符串并使用sha256加密 加密后再使用base64加密
时间: 2024-07-24 12:00:52 浏览: 200
在Java中,你可以通过以下步骤生成随机字符串,然后使用SHA-256算法进行加密,最后对加密结果再使用Base64编码:
1. **生成随机字符串**:
```java
import java.util.Random;
import java.security.SecureRandom;
public String generateRandomString(int length) {
SecureRandom random = new SecureRandom();
StringBuilder sb = new StringBuilder(length);
for (int i = 0; i < length; i++) {
int randomChar = random.nextInt(97 + 26); // 随机字符范围,包括小写字母a-z
sb.append((char) (randomChar));
}
return sb.toString();
}
```
2. **使用SHA-256加密**:
```java
import javax.crypto.Mac;
import javax.crypto.spec.SecretKeySpec;
import java.nio.charset.StandardCharsets;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
public String sha256Hash(String input) throws NoSuchAlgorithmException, InvalidKeyException {
SecretKeySpec key = new SecretKeySpec("your-secret-key".getBytes(), "HmacSHA256");
Mac mac = Mac.getInstance("HmacSHA256");
mac.init(key);
byte[] hashBytes = mac.doFinal(input.getBytes(StandardCharsets.UTF_8));
return Base64.getEncoder().encodeToString(hashBytes);
}
```
这里假设有一个"your-secret-key"作为你的密钥,你需要替换为你实际使用的密钥。
3. **两次加密后的Base64编码**:
```java
public String doubleEncrypt(String input) {
String intermediateHash = sha256Hash(input);
return Base64.getEncoder().encodeToString(intermediateHash.getBytes());
}
```
现在你可以先生成随机字符串,然后通过`doubleEncrypt`函数获取双层加密的Base64字符串。
阅读全文
相关推荐
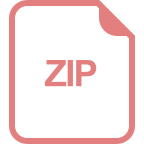
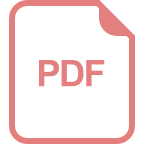
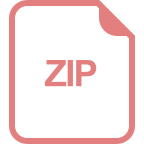















