识别车牌号的机器学习源代码
时间: 2023-08-24 15:06:14 浏览: 59
识别车牌号的机器学习源代码通常包括以下步骤:
1. 数据收集:收集大量的车牌图片,包括各种不同的车牌类型和不同的光照条件下的图片。
2. 数据预处理:对图片进行预处理,包括图像增强、图像切割和图像大小归一化等操作。
3. 特征提取:使用特征提取算法提取图片中的特征,常用的算法包括HOG、LBP和Haar等。
4. 训练模型:使用机器学习算法训练模型,常用的算法包括SVM、神经网络和决策树等。
5. 测试模型:使用测试数据对模型进行测试,评估模型的性能。
以下是一个使用Python实现的车牌识别代码示例:
```python
import cv2
import numpy as np
import pytesseract
# 预处理函数
def preprocess(img):
# 灰度化
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 二值化
ret, thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)
# 去除图像噪声
kernel = np.ones((3, 3), np.uint8)
opening = cv2.morphologyEx(thresh, cv2.MORPH_OPEN, kernel, iterations=2)
# 图像膨胀
dilate = cv2.dilate(opening, kernel, iterations=1)
return dilate
# 车牌识别函数
def recognize_plate(img):
# 预处理图像
processed_img = preprocess(img)
# 查找轮廓
contours, hierarchy = cv2.findContours(processed_img, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# 找到最大的轮廓
max_cnt = max(contours, key=cv2.contourArea)
# 获取最小矩形框
rect = cv2.minAreaRect(max_cnt)
# 获取矩形四个顶点坐标
box = cv2.boxPoints(rect)
box = np.int0(box)
# 透视变换
width = int(rect[1][0])
height = int(rect[1][1])
src_pts = box.astype("float32")
dst_pts = np.array([[0, height - 1], [0, 0], [width - 1, 0], [width - 1, height - 1]], dtype="float32")
M = cv2.getPerspectiveTransform(src_pts, dst_pts)
warped = cv2.warpPerspective(img, M, (width, height))
# OCR识别
gray = cv2.cvtColor(warped, cv2.COLOR_BGR2GRAY)
result = pytesseract.image_to_string(gray, lang='chi_sim')
return result
# 加载测试图片并进行车牌识别
img = cv2.imread('test.jpg')
result = recognize_plate(img)
print(result)
```
需要注意的是,该代码示例中使用了Python的OpenCV库和Pytesseract库来实现车牌识别功能。其中,OpenCV库用于图像处理和轮廓查找,Pytesseract库用于OCR识别。
相关推荐
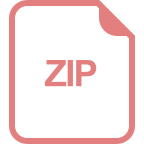
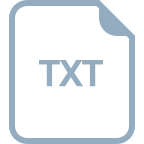
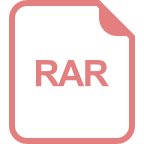










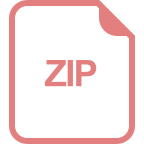
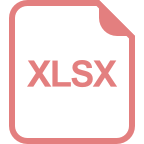