c语言 替换文件中的字符,C语言文件中字符串的查找与替换?
时间: 2023-11-28 19:50:40 浏览: 111
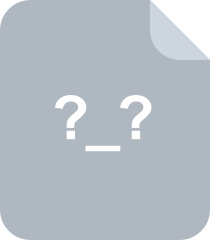
打开文档,字符串的查找与替换,保存 C语言

可以使用C语言中的文件操作函数和字符串处理函数来实现替换文件中的字符和字符串。
首先,使用fopen函数打开要操作的文件,并指定打开方式为“读写”或“只写”。然后,使用fgets函数逐行读取文件内容,对每行内容进行字符串处理,查找需要替换的字符或字符串,使用strcpy和strcat函数将替换后的字符串写入到一个新的文件中。最后,使用fclose函数关闭文件。
以下是一个简单的示例代码,用于替换文件中的字符:
```c
#include <stdio.h>
#include <string.h>
int main()
{
FILE *fp1, *fp2;
char c, old_char, new_char;
char filename[100];
// 输入文件名和需要替换的字符
printf("Enter the filename: ");
scanf("%s", filename);
printf("Enter the character to replace: ");
scanf(" %c", &old_char);
printf("Enter the new character: ");
scanf(" %c", &new_char);
// 打开文件
fp1 = fopen(filename, "r");
fp2 = fopen("temp.txt", "w");
// 逐个字符读取文件内容,替换需要替换的字符并写入到新文件中
while ((c = fgetc(fp1)) != EOF)
{
if (c == old_char)
{
fputc(new_char, fp2);
}
else
{
fputc(c, fp2);
}
}
// 关闭文件
fclose(fp1);
fclose(fp2);
// 删除原文件并将新文件重命名为原文件名
remove(filename);
rename("temp.txt", filename);
printf("Replacement completed!\n");
return 0;
}
```
如果需要替换字符串,可以使用strstr函数查找需要替换的字符串,并使用strcpy和strcat函数将替换后的字符串写入到新的文件中。
阅读全文
相关推荐
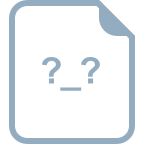
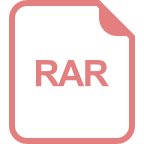
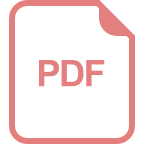
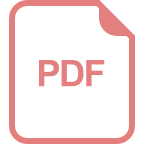
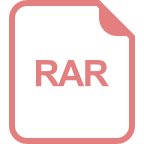
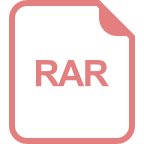
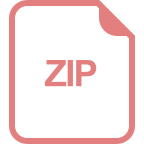
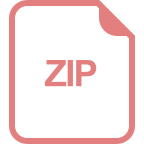
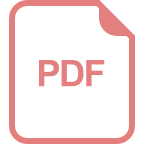
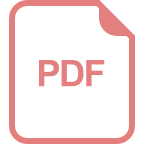
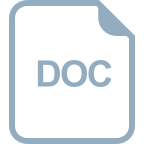