写一段mybatisplus框架的crud代码
时间: 2023-09-16 17:05:35 浏览: 151
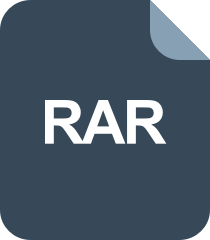
使用mybatis实现CRUD
### 回答1:
好的,以下是一段使用 MybatisPlus 框架进行 CRUD 操作的示例代码:
// 导入必要的类
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;
// 定义实体类
public class User {
private Long id;
private String name;
private Integer age;
// 省略 getter 和 setter 方法
}
// 定义 Mapper 接口
public interface UserMapper extends BaseMapper<User> {}
// 定义 Service 类
@Service
public class UserService extends ServiceImpl<UserMapper, User> {}
// 在 Controller 中使用 Service 进行 CRUD 操作
@RestController
@RequestMapping("/users")
public class UserController {
@Autowired
private UserService userService;
// 查询所有用户
@GetMapping("/")
public List<User> list() {
return userService.list();
}
// 根据 ID 查询用户
@GetMapping("/{id}")
public User getById(@PathVariable Long id) {
return userService.getById(id);
}
// 新增用户
@PostMapping("/")
public boolean save(@RequestBody User user) {
return userService.save(user);
}
// 更新用户
@PutMapping("/{id}")
public boolean updateById(@PathVariable Long id, @RequestBody User user) {
user.setId(id);
return userService.updateById(user);
}
// 根据 ID 删除用户
@DeleteMapping("/{id}")
public boolean removeById(@PathVariable Long id) {
return userService.removeById(id);
}
// 根据条件查询用户
@GetMapping("/search")
public List<User> search(String name, Integer age) {
QueryWrapper<User> queryWrapper = new QueryWrapper<>();
if (name != null) {
queryWrapper.like("name", name);
}
if (age != null) {
queryWrapper.eq("age", age);
}
return userService.list(queryWrapper);
}
}
希望这段代码能够帮助到你!
### 回答2:
MyBatis Plus是一个基于MyBatis的ORM框架,提供了更加简洁方便的CRUD操作方法。下面是使用MyBatis Plus进行CRUD操作的代码示例:
1. 添加依赖
首先,在项目的pom.xml文件中添加MyBatis Plus的依赖:
```xml
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>最新版本号</version>
</dependency>
```
2. 创建实体类
创建对应数据库表的实体类,并使用注解标注相关信息(例如表名、字段等):
```java
@Data
@TableName("user")
public class User {
@TableId(type = IdType.AUTO)
private Long id;
private String name;
private Integer age;
// 省略getter和setter方法
}
```
3. 创建Mapper接口
创建对应实体类的Mapper接口,并继承BaseMapper接口,无需实现任何方法:
```java
public interface UserMapper extends BaseMapper<User> {
}
```
4. 使用CRUD操作
在需要进行CRUD操作的地方,通过依赖注入的方式注入UserMapper,并调用相应的方法进行CRUD操作:
```java
@Autowired
private UserMapper userMapper;
// 添加用户
public void addUser(User user) {
userMapper.insert(user);
}
// 更新用户
public void updateUser(User user) {
userMapper.updateById(user);
}
// 删除用户
public void deleteUser(Long id) {
userMapper.deleteById(id);
}
// 查询用户
public User getUserById(Long id) {
return userMapper.selectById(id);
}
```
以上就是使用MyBatis Plus进行CRUD操作的示例代码。通过MyBatis Plus框架的简洁方法,我们可以更加方便地进行数据库的增删改查操作。
### 回答3:
MyBatis Plus是一个基于MyBatis的增强框架,简化了数据库操作的CRUD代码。下面是一个使用MyBatis Plus进行CRUD操作的示例代码。
首先,我们需要在配置文件中配置MyBatis Plus的基本信息,如数据库连接信息、数据库方言等。接下来,我们创建实体类,用于映射数据库表。
```java
// 导入所需的包
import com.baomidou.mybatisplus.annotation.*;
import lombok.Data;
// 声明实体类
@Data
@TableName("user")
public class User {
@TableId(type = IdType.AUTO)
private Long id;
private String name;
private Integer age;
private String email;
}
```
在实现CRUD操作之前,我们需要创建对应的Mapper接口,继承自MyBatis Plus提供的BaseMapper接口。
```java
// 导入所需的包
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import org.apache.ibatis.annotations.Mapper;
// 声明Mapper接口
@Mapper
public interface UserMapper extends BaseMapper<User> {
}
```
现在可以进行CRUD操作了。
1. 创建记录:
```java
// 导入所需的包
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
// 注入Mapper接口实例
@Service
public class UserService {
@Autowired
private UserMapper userMapper;
public void createUser(User user) {
userMapper.insert(user);
}
}
```
2. 查询记录:
```java
// 导入所需的包
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
// 注入Mapper接口实例
@Service
public class UserService {
@Autowired
private UserMapper userMapper;
public User getUserById(Long id) {
return userMapper.selectById(id);
}
}
```
3. 更新记录:
```java
// 导入所需的包
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
// 注入Mapper接口实例
@Service
public class UserService {
@Autowired
private UserMapper userMapper;
public void updateUser(User user) {
userMapper.updateById(user);
}
}
```
4. 删除记录:
```java
// 导入所需的包
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
// 注入Mapper接口实例
@Service
public class UserService {
@Autowired
private UserMapper userMapper;
public void deleteUserById(Long id) {
userMapper.deleteById(id);
}
}
```
以上就是使用MyBatis Plus进行CRUD操作的示例代码。通过引入MyBatis Plus框架,我们可以简化数据库操作的代码,提高开发效率。
阅读全文
相关推荐
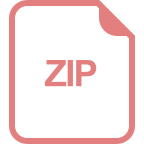
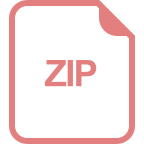
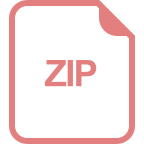
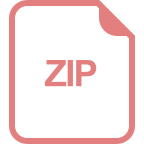
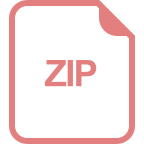
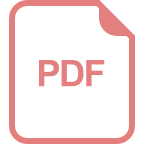
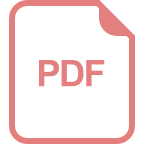
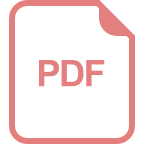
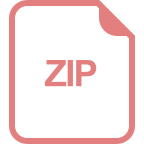
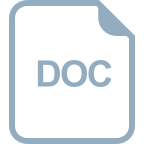
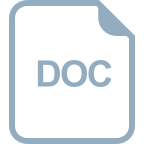



