结构体冒泡排序降序c语言
时间: 2023-10-11 12:13:35 浏览: 45
以下是结构体冒泡排序降序的 C 语言代码示例:
```c
#include <stdio.h>
#include <string.h>
#define MAX_SIZE 100
struct Student {
char name[20];
int score;
};
void bubbleSort(struct Student arr[], int n);
int main() {
struct Student arr[MAX_SIZE];
int n, i;
printf("Enter the number of students: ");
scanf("%d", &n);
printf("Enter the name and score of each student:\n");
for (i = 0; i < n; i++) {
scanf("%s %d", arr[i].name, &arr[i].score);
}
bubbleSort(arr, n);
printf("Sorted list of students (in descending order of score):\n");
for (i = 0; i < n; i++) {
printf("%s %d\n", arr[i].name, arr[i].score);
}
return 0;
}
void bubbleSort(struct Student arr[], int n) {
int i, j;
struct Student temp;
for (i = 0; i < n - 1; i++) {
for (j = 0; j < n - i - 1; j++) {
if (arr[j].score < arr[j + 1].score) {
// Swap the elements
temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
```
在上面的代码中,我们定义了一个名为 `Student` 的结构体,它包含了一个学生的姓名和分数。我们使用 `scanf` 函数从用户输入中读取每个学生的姓名和分数,并将它们存储在结构体数组中。然后,我们调用 `bubbleSort` 函数对结构体数组进行降序排序,最后输出排序后的结果。
`bubbleSort` 函数使用冒泡排序算法对结构体数组进行排序。它使用两个嵌套的循环来比较相邻的元素,并根据需要交换它们的位置。在每次迭代中,最大的元素都会“浮”到数组的顶部。由于我们要按照分数的降序排序,因此比较时使用 `<` 运算符而不是 `>` 运算符。
阅读全文
相关推荐
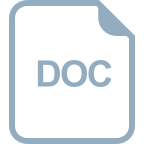




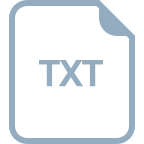
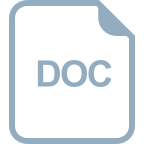
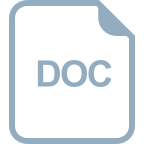
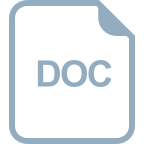



