hadoop编程首先利用编程将给定文件的数据读入HBase表中。 利用编程从HBASE中读取学生成绩,并实现计算每个学生成绩平均分和每门课程成绩平均分的功能,要求对其中的主要代码进行注释性解释,然后在HDFS中查询程序运行结果。
时间: 2024-02-23 14:02:01 浏览: 222
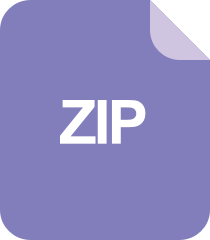
用于计算学生的平均成绩,采用的平台是hadoop分布式平台
好的,这是您的第一个问题。让我来回答。
首先,我们需要编写一个程序将给定文件的数据读入HBase表中。这可以通过使用HBase的Java API来完成。下面是一个示例代码:
```java
Configuration config = HBaseConfiguration.create();
Connection connection = ConnectionFactory.createConnection(config);
TableName tableName = TableName.valueOf("students");
Table table = connection.getTable(tableName);
BufferedReader reader = new BufferedReader(new FileReader("students.txt"));
String line = reader.readLine();
while (line != null) {
String[] parts = line.split(",");
Put put = new Put(Bytes.toBytes(parts[0]));
put.addColumn(Bytes.toBytes("info"), Bytes.toBytes("name"), Bytes.toBytes(parts[1]));
put.addColumn(Bytes.toBytes("info"), Bytes.toBytes("score"), Bytes.toBytes(parts[2]));
table.put(put);
line = reader.readLine();
}
reader.close();
table.close();
connection.close();
```
上述代码创建了一个HBase配置对象,并使用它来创建一个连接对象。接着,我们定义了一个表名,并使用连接对象获取该表的实例。然后,我们使用Java的IO库读取给定的文件,并将每行数据按照逗号分隔为三个部分。然后,我们创建一个Put对象来存储每行数据,并将其添加到表中。
下一步是从HBase中读取学生成绩,并实现计算每个学生成绩平均分和每门课程成绩平均分的功能。这也可以通过使用HBase的Java API来完成。下面是一个示例代码:
```java
Configuration config = HBaseConfiguration.create();
Connection connection = ConnectionFactory.createConnection(config);
TableName tableName = TableName.valueOf("students");
Table table = connection.getTable(tableName);
Scan scan = new Scan();
ResultScanner scanner = table.getScanner(scan);
Map<String, List<Integer>> studentScores = new HashMap<>();
Map<String, List<Integer>> courseScores = new HashMap<>();
for (Result result : scanner) {
String studentId = Bytes.toString(result.getRow());
String studentName = Bytes.toString(result.getValue(Bytes.toBytes("info"), Bytes.toBytes("name")));
int score = Bytes.toInt(result.getValue(Bytes.toBytes("info"), Bytes.toBytes("score")));
if (!studentScores.containsKey(studentId)) {
studentScores.put(studentId, new ArrayList<>());
}
studentScores.get(studentId).add(score);
if (!courseScores.containsKey(studentName)) {
courseScores.put(studentName, new ArrayList<>());
}
courseScores.get(studentName).add(score);
}
for (String studentId : studentScores.keySet()) {
List<Integer> scores = studentScores.get(studentId);
int sum = 0;
for (int score : scores) {
sum += score;
}
double avg = (double) sum / scores.size();
System.out.println("Student " + studentId + " average score: " + avg);
}
for (String courseName : courseScores.keySet()) {
List<Integer> scores = courseScores.get(courseName);
int sum = 0;
for (int score : scores) {
sum += score;
}
double avg = (double) sum / scores.size();
System.out.println("Course " + courseName + " average score: " + avg);
}
scanner.close();
table.close();
connection.close();
```
上述代码创建了一个HBase配置对象,并使用它来创建一个连接对象。接着,我们定义了一个表名,并使用连接对象获取该表的实例。然后,我们使用Scan对象来扫描表中的所有数据,并使用ResultScanner对象来获取扫描结果。
接下来,我们定义了两个Map对象来存储学生分数和课程分数。我们使用Java的字节数组工具类Bytes来获取每一行数据的行键、学生姓名和分数,并将它们添加到相应的Map对象中。
最后,我们在两个Map对象上执行循环,计算每个学生的平均分和每门课程的平均分,并将结果打印出来。
最后,在HDFS中查询程序运行结果,可以使用Hadoop的命令行工具来完成。下面是一个示例命令:
```
hadoop fs -cat /output/part-r-00000
```
其中,/output/part-r-00000是程序输出的结果文件路径。
阅读全文
相关推荐
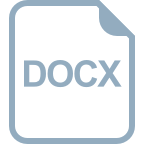
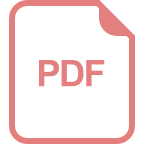
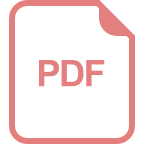
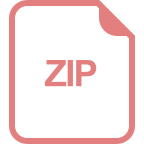
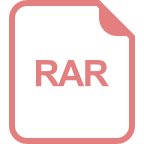
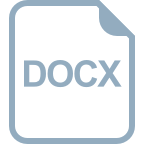
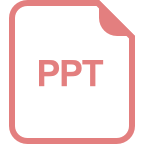
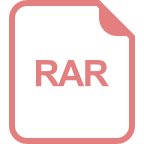
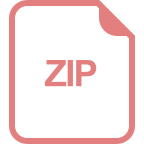
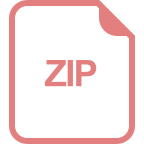
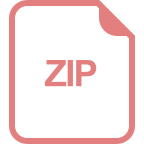
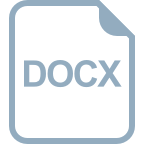
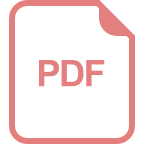
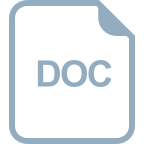
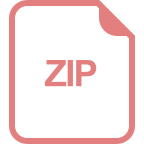
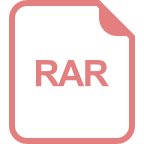