JavaScript常用内置对象使用例题
时间: 2023-07-22 21:13:30 浏览: 132
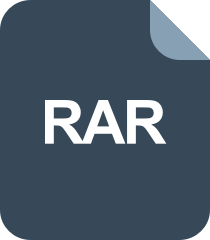
jsp内置对象的应用范例
好的,下面是几个常用内置对象的使用例题:
1. 字符串对象
```js
let str = "hello world";
// 获取字符串长度
console.log(str.length); // 11
// 转换为大写字母
console.log(str.toUpperCase()); // "HELLO WORLD"
// 替换字符串
console.log(str.replace("world", "JavaScript")); // "hello JavaScript"
```
2. 数组对象
```js
let arr = [1, 2, 3, 4, 5];
// 获取数组长度
console.log(arr.length); // 5
// 获取数组中第一个元素
console.log(arr[0]); // 1
// 循环遍历数组
for (let i = 0; i < arr.length; i++) {
console.log(arr[i]);
}
// 在数组末尾添加一个元素
arr.push(6);
console.log(arr); // [1, 2, 3, 4, 5, 6]
// 删除数组中最后一个元素
arr.pop();
console.log(arr); // [1, 2, 3, 4, 5]
```
3. Date对象
```js
let now = new Date();
// 获取当前时间
console.log(now); // Sat Jul 10 2021 14:32:06 GMT+0800 (中国标准时间)
// 获取当前时间的年份
console.log(now.getFullYear()); // 2021
// 获取当前时间的月份(0表示1月)
console.log(now.getMonth()); // 6
// 获取当前时间的日期
console.log(now.getDate()); // 10
```
4. Math对象
```js
// 获取随机数(0-1之间)
console.log(Math.random()); // 0.123456789
// 获取一个数的绝对值
console.log(Math.abs(-10)); // 10
// 获取两个数的最大值
console.log(Math.max(1, 2, 3, 4, 5)); // 5
```
以上只是一些常用内置对象的例题,JavaScript内置对象非常多,不同的对象有不同的属性和方法,需要根据实际需求灵活运用。
阅读全文
相关推荐
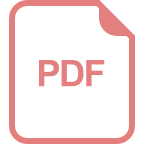
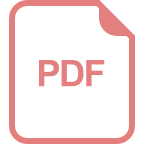
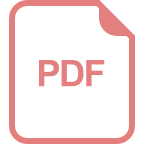
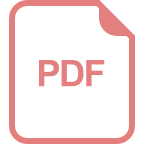
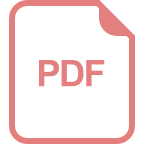
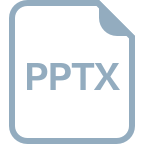
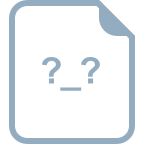
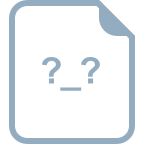
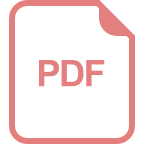
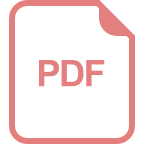
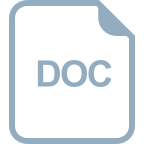
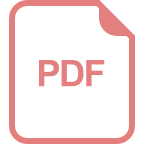
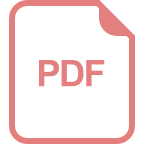
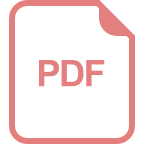
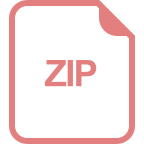