3维粒子群算法matlab
时间: 2023-09-24 20:09:46 浏览: 124
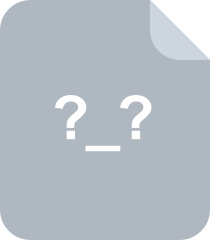
三维粒子群算法
以下是一个简单的3维粒子群算法的MATLAB代码示例:
```matlab
% 3D Particle Swarm Optimization
% Define the objective function
fun = @(x) 100*(x(2)-x(1)^2)^2 + (1-x(1))^2 + 100*(x(3)-x(2)^2)^2 + (1-x(2))^2;
% Define the lower and upper bounds of the variables
lb = [-5,-5,-5];
ub = [5,5,5];
% Define the number of particles and iterations
nParticles = 50;
nIterations = 100;
% Initialize the particle positions and velocities
particlePositions = bsxfun(@plus,lb,bsxfun(@times,rand(nParticles,3),ub-lb));
particleVelocities = zeros(nParticles,3);
% Initialize the personal best positions and values
personalBestPositions = particlePositions;
personalBestValues = inf(nParticles,1);
% Initialize the global best position and value
globalBestPosition = zeros(1,3);
globalBestValue = inf;
% Run the main loop
for iIteration = 1:nIterations
% Evaluate the objective function for each particle
particleValues = arrayfun(fun,particlePositions);
% Update the personal best positions and values
needToUpdatePersonalBest = particleValues < personalBestValues;
personalBestPositions(needToUpdatePersonalBest,:) = particlePositions(needToUpdatePersonalBest,:);
personalBestValues(needToUpdatePersonalBest) = particleValues(needToUpdatePersonalBest);
% Update the global best position and value
[iterationBestValue,iterationBestIndex] = min(personalBestValues);
if iterationBestValue < globalBestValue
globalBestPosition = personalBestPositions(iterationBestIndex,:);
globalBestValue = iterationBestValue;
end
% Update the particle velocities and positions
inertiaWeight = 0.729; % Inertia weight
cognitiveWeight = 1.49445; % Cognitive weight
socialWeight = 1.49445; % Social weight
r1 = rand(nParticles,3); % Random numbers
r2 = rand(nParticles,3); % Random numbers
cognitiveComponent = bsxfun(@times,cognitiveWeight*r1,bsxfun(@minus,personalBestPositions,particlePositions));
socialComponent = bsxfun(@times,socialWeight*r2,bsxfun(@minus,globalBestPosition,particlePositions));
particleVelocities = bsxfun(@plus,inertiaWeight*particleVelocities,cognitiveComponent+socialComponent);
particlePositions = particlePositions + particleVelocities;
% Enforce the lower and upper bounds of the variables
particlePositions = max(particlePositions,lb);
particlePositions = min(particlePositions,ub);
end
% Display the global best position and value
disp(['Global best position: ',num2str(globalBestPosition)]);
disp(['Global best value: ',num2str(globalBestValue)]);
```
这个代码实现了一个简单的3D粒子群算法来最小化一个测试函数。你可以将函数`fun`替换成你自己的目标函数,并调整粒子数量、迭代次数、变量的上下限等参数来适应你的问题。
阅读全文
相关推荐

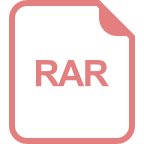
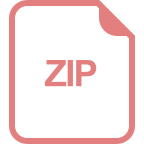
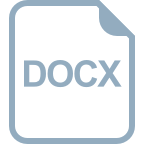
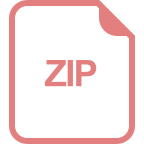
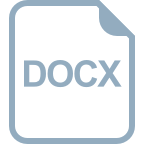
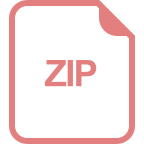
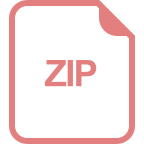
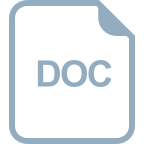
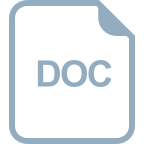
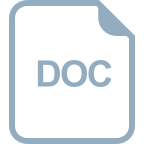




