@app.post("/register") async def fastapi_register(request:Request): content_type = request.headers.get("Content-Type") if content_type != "application/json": return {"error": "Invalid Content-Type"} try: # 如果无法解析为JSON,会抛出异常 json_data = await request.json() except json.JSONDecodeError: return {"error": "Invalid JSON"} return {"data": json_data},获取不到数据
时间: 2023-07-19 18:36:26 浏览: 60
在你的代码中,如果请求体中的 Content-Type 不是 JSON 类型,你返回了一个带有错误信息的字典对象。这是正确的做法,因为如果客户端发送的请求格式不正确,应该返回一个错误响应。
但是,在请求格式正确的情况下,你在返回时却遇到了问题。具体来说,你使用了 `await request.json()` 来解析请求体中的 JSON 数据,但是你没有检查这个方法的返回值,也没有使用 `try...except` 来处理解析错误的情况。因此,如果请求体中的 JSON 数据无法解析,你的代码就会抛出异常,从而导致无法正确返回数据。
为了解决这个问题,你可以使用如下的代码来处理请求体中的 JSON 数据,并在解析错误时返回错误响应:
```python
import json
from fastapi import FastAPI, Request
from fastapi.responses import JSONResponse
app = FastAPI()
@app.post("/register")
async def register(request: Request):
content_type = request.headers.get("Content-Type")
if content_type != "application/json":
return JSONResponse(content={"error": "Invalid Content-Type"})
try:
json_data = await request.json()
except json.JSONDecodeError:
return JSONResponse(content={"error": "Invalid JSON"})
return JSONResponse(content={"data": json_data})
```
在上述代码中,我们首先检查请求体的 Content-Type 是否为 application/json,如果不是,则返回一个错误响应。接着,我们使用 `try...except` 来捕获解析 JSON 数据时可能出现的异常,如果出现异常,则返回一个带有错误信息的 JSON 响应。
最后,如果解析成功,我们返回一个带有解析后 JSON 数据的 JSON 响应。
注意,我们在返回 JSON 响应时,使用了 FastAPI 框架提供的 `JSONResponse` 类,而不是普通的字典对象。这是因为 `JSONResponse` 类会自动将响应体中的 Python 对象序列化为 JSON 格式,从而方便客户端的处理。
相关推荐
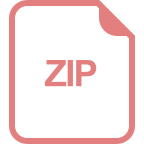















