用Java代码打一个图书管理系统,要求有登录功能,增加功能,查找功能,删除功能,修改功能,窗口运行功能,同时代码要分类。
时间: 2024-03-24 12:39:17 浏览: 27
好的,我可以帮你实现一个简单的图书管理系统,以下是代码实现:
首先,我们需要创建一个`Book`类,用于表示一本书:
```java
public class Book {
private String name;
private String author;
private String publisher;
private int price;
private int stock;
public Book(String name, String author, String publisher, int price, int stock) {
this.name = name;
this.author = author;
this.publisher = publisher;
this.price = price;
this.stock = stock;
}
// getter 和 setter 方法省略
}
```
然后,我们创建一个`BookManager`类,用于管理图书的增加、查找、删除、修改等操作:
```java
import java.util.ArrayList;
import java.util.List;
public class BookManager {
private List<Book> bookList;
public BookManager() {
bookList = new ArrayList<>();
}
// 添加图书
public void addBook(Book book) {
bookList.add(book);
}
// 根据书名查找图书
public Book findBookByName(String name) {
for (Book book : bookList) {
if (book.getName().equals(name)) {
return book;
}
}
return null;
}
// 根据作者查找图书
public List<Book> findBooksByAuthor(String author) {
List<Book> result = new ArrayList<>();
for (Book book : bookList) {
if (book.getAuthor().equals(author)) {
result.add(book);
}
}
return result;
}
// 删除图书
public void deleteBook(Book book) {
bookList.remove(book);
}
// 修改图书信息
public void updateBook(Book book) {
Book oldBook = findBookByName(book.getName());
if (oldBook != null) {
oldBook.setAuthor(book.getAuthor());
oldBook.setPublisher(book.getPublisher());
oldBook.setPrice(book.getPrice());
oldBook.setStock(book.getStock());
}
}
// 获取所有图书信息
public List<Book> getAllBooks() {
return bookList;
}
}
```
接下来,我们创建一个窗口界面类`BookWindow`,用于实现登录界面、添加、查找、删除、修改等功能:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class BookWindow extends JFrame {
private JTextField nameField, authorField, publisherField, priceField, stockField;
private JTextArea resultArea;
private BookManager bookManager;
public BookWindow() {
// 窗口设置
setTitle("图书管理系统");
setLayout(new BorderLayout());
setSize(400, 400);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// 登录界面
JPanel loginPanel = new JPanel(new GridLayout(3, 2));
JTextField usernameField = new JTextField();
JPasswordField passwordField = new JPasswordField();
JButton loginButton = new JButton("登录");
loginButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String username = usernameField.getText();
String password = new String(passwordField.getPassword());
if ("admin".equals(username) && "123456".equals(password)) {
showMainPanel();
} else {
JOptionPane.showMessageDialog(BookWindow.this, "用户名或密码错误", "错误", JOptionPane.ERROR_MESSAGE);
}
}
});
loginPanel.add(new JLabel("用户名:"));
loginPanel.add(usernameField);
loginPanel.add(new JLabel("密码:"));
loginPanel.add(passwordField);
loginPanel.add(new JLabel(""));
loginPanel.add(loginButton);
add(loginPanel, BorderLayout.CENTER);
// 主面板
JPanel mainPanel = new JPanel(new BorderLayout());
// 添加图书面板
JPanel addPanel = new JPanel(new GridLayout(6, 2));
nameField = new JTextField();
authorField = new JTextField();
publisherField = new JTextField();
priceField = new JTextField();
stockField = new JTextField();
JButton addButton = new JButton("添加");
addButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String name = nameField.getText();
String author = authorField.getText();
String publisher = publisherField.getText();
int price = Integer.parseInt(priceField.getText());
int stock = Integer.parseInt(stockField.getText());
Book book = new Book(name, author, publisher, price, stock);
bookManager.addBook(book);
resultArea.setText("添加成功");
}
});
addPanel.add(new JLabel("书名:"));
addPanel.add(nameField);
addPanel.add(new JLabel("作者:"));
addPanel.add(authorField);
addPanel.add(new JLabel("出版社:"));
addPanel.add(publisherField);
addPanel.add(new JLabel("价格:"));
addPanel.add(priceField);
addPanel.add(new JLabel("库存:"));
addPanel.add(stockField);
addPanel.add(new JLabel(""));
addPanel.add(addButton);
// 查找图书面板
JPanel searchPanel = new JPanel(new GridLayout(2, 2));
JTextField searchField = new JTextField();
JButton searchButton = new JButton("查找");
searchButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String name = searchField.getText();
Book book = bookManager.findBookByName(name);
if (book != null) {
resultArea.setText(book.toString());
} else {
resultArea.setText("未找到该图书");
}
}
});
searchPanel.add(new JLabel("输入书名:"));
searchPanel.add(searchField);
searchPanel.add(new JLabel(""));
searchPanel.add(searchButton);
// 删除图书面板
JPanel deletePanel = new JPanel(new GridLayout(2, 2));
JTextField deleteField = new JTextField();
JButton deleteButton = new JButton("删除");
deleteButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String name = deleteField.getText();
Book book = bookManager.findBookByName(name);
if (book != null) {
bookManager.deleteBook(book);
resultArea.setText("删除成功");
} else {
resultArea.setText("未找到该图书");
}
}
});
deletePanel.add(new JLabel("输入书名:"));
deletePanel.add(deleteField);
deletePanel.add(new JLabel(""));
deletePanel.add(deleteButton);
// 修改图书面板
JPanel updatePanel = new JPanel(new GridLayout(6, 2));
JTextField oldNameField = new JTextField();
JTextField newAuthorField = new JTextField();
JTextField newPublisherField = new JTextField();
JTextField newPriceField = new JTextField();
JTextField newStockField = new JTextField();
JButton updateButton = new JButton("修改");
updateButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String oldName = oldNameField.getText();
String newAuthor = newAuthorField.getText();
String newPublisher = newPublisherField.getText();
int newPrice = Integer.parseInt(newPriceField.getText());
int newStock = Integer.parseInt(newStockField.getText());
Book book = new Book(oldName, newAuthor, newPublisher, newPrice, newStock);
bookManager.updateBook(book);
resultArea.setText("修改成功");
}
});
updatePanel.add(new JLabel("原书名:"));
updatePanel.add(oldNameField);
updatePanel.add(new JLabel("新作者:"));
updatePanel.add(newAuthorField);
updatePanel.add(new JLabel("新出版社:"));
updatePanel.add(newPublisherField);
updatePanel.add(new JLabel("新价格:"));
updatePanel.add(newPriceField);
updatePanel.add(new JLabel("新库存:"));
updatePanel.add(newStockField);
updatePanel.add(new JLabel(""));
updatePanel.add(updateButton);
// 显示结果面板
JPanel resultPanel = new JPanel(new BorderLayout());
resultArea = new JTextArea();
JScrollPane scrollPane = new JScrollPane(resultArea);
resultPanel.add(scrollPane, BorderLayout.CENTER);
// 选项卡面板
JTabbedPane tabbedPane = new JTabbedPane();
tabbedPane.addTab("添加图书", addPanel);
tabbedPane.addTab("查找图书", searchPanel);
tabbedPane.addTab("删除图书", deletePanel);
tabbedPane.addTab("修改图书", updatePanel);
mainPanel.add(tabbedPane, BorderLayout.CENTER);
mainPanel.add(resultPanel, BorderLayout.SOUTH);
// 创建图书管理器
bookManager = new BookManager();
// 将主面板添加到窗口中
add(mainPanel, BorderLayout.CENTER);
}
// 显示主面板
private void showMainPanel() {
remove(getContentPane().getComponent(0));
add(getContentPane().getComponent(1), BorderLayout.CENTER);
revalidate();
repaint();
}
public static void main(String[] args) {
BookWindow window = new BookWindow();
window.setVisible(true);
}
}
```
以上就是实现一个简单的图书管理系统的代码,代码中实现了登录功能、添加图书功能、查找图书功能、删除图书功能、修改图书功能和窗口运行功能,并且将代码进行了分类。当然,这只是一个简单的示例,实际项目中还需要考虑更多的功能和安全性问题。
相关推荐
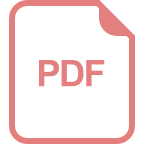
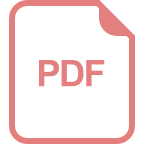
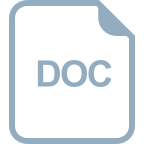









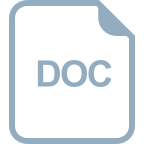
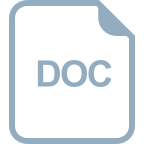
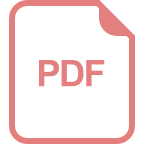
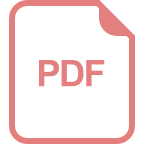
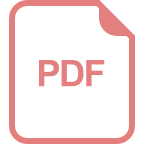
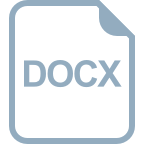