使用运行时API定位内存泄露
时间: 2023-10-08 16:03:48 浏览: 43
使用运行时API可以定位内存泄露,具体可以通过以下步骤实现:
1. 在 Xcode 中选择 Product -> Scheme -> Edit Scheme,然后在左侧选择 Run,接着在 Arguments Passed On Launch 中添加环境变量:MallocStackLoggingNoCompact,并将其值设为 1。
2. 在代码中添加以下代码:
```
#import <objc/runtime.h>
void checkMemoryLeak() {
unsigned int count = 0;
Class *classes = objc_copyClassList(&count);
for (unsigned int i = 0; i < count; i++) {
Class cls = classes[i];
if (class_respondsToSelector(cls, @selector(doesNotRecognizeSelector:))) {
// This class has no method implementations of its own.
continue;
}
unsigned int methodCount = 0;
Method *methods = class_copyMethodList(cls, &methodCount);
for (unsigned int j = 0; j < methodCount; j++) {
Method method = methods[j];
SEL selector = method_getName(method);
if (selector == @selector(alloc) || selector == @selector(new)) {
// Skip alloc and new methods to avoid false positives.
continue;
}
NSString *methodName = NSStringFromSelector(selector);
if ([methodName hasPrefix:@"init"]) {
// Skip init methods to avoid false positives.
continue;
}
IMP imp = method_getImplementation(method);
if (imp == NULL) {
// Skip methods with no implementation.
continue;
}
if (strstr(methodName.UTF8String, "copy") != NULL || strstr(methodName.UTF8String, "mutableCopy") != NULL) {
// Skip copy and mutableCopy methods to avoid false positives.
continue;
}
if (strstr(methodName.UTF8String, "retain") != NULL) {
// Skip retain methods to avoid false positives.
continue;
}
if (strstr(methodName.UTF8String, "release") != NULL || strstr(methodName.UTF8String, "autorelease") != NULL) {
// Skip release and autorelease methods to avoid false positives.
continue;
}
// Call the method with a nil argument to create an object without retaining it.
((id (*)(id, SEL, ...))method_invoke)(cls, selector, nil);
}
free(methods);
}
free(classes);
}
```
3. 在代码中调用 checkMemoryLeak 函数,这个函数会递归遍历所有类及其方法,并在不保留对象的情况下调用方法,从而创建对象并触发内存分配。如果有内存泄漏,可以通过 Instruments 工具捕获堆栈信息并进行分析。
需要注意的是,这个方法只是一种定位内存泄漏的手段,具体的问题还需要根据具体情况进行分析和解决。
相关推荐
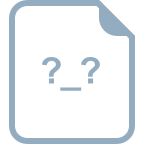
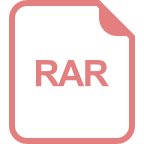
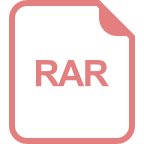
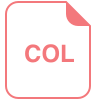
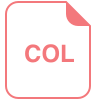
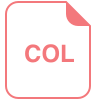
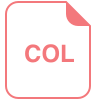
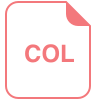








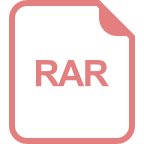
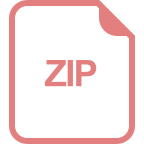