import os import cv2 from PIL import Image, ImageDraw, ImageFont import numpy as np # 输入文本 text = "这是一个跑马灯视频" # 设置字体 font_size = 1000 font_path = "msyh.ttc" font = ImageFont.truetype(font_path, font_size) # 获取文本宽度 img = Image.new('RGB', (1, 1), color=(255, 255, 255)) draw = ImageDraw.Draw(img) text_width, text_height = draw.textsize(text, font) # 设置视频参数 width = text_width + 100 height = text_height + 50 fps = 60 seconds = 10 frames = fps * seconds # 创建视频 fourcc = cv2.VideoWriter_fourcc(*'mp4v') output_path = os.path.join("E:\Template\word", "output.mp4") video = cv2.VideoWriter(output_path, fourcc, fps, (width, height)) # 生成每一帧的图像 for i in range(frames): # 创建背景 background = Image.new('RGB', (width, height), color=(255, 0, 0)) # 创建文本 text_image = Image.new('RGB', (text_width, text_height), color=(255, 0, 0)) draw = ImageDraw.Draw(text_image) draw.text((0, 0), text, font=font, fill=(255, 255, 255)) # 将文本贴到背景上 x_offset = (i - frames) * (text_width + 100) // frames background.paste(text_image, (x_offset, 25)) # 将图像转换为视频帧 frame = cv2.cvtColor(np.array(background), cv2.COLOR_RGB2BGR) video.write(frame) # 释放视频 video.release() 帮我写优化python代码 根据文本生成跑马灯视频 红色背景 白色文本 视频1080P分辨率1920*1080 文本从右往左滚动
时间: 2024-01-27 09:04:21 浏览: 164
import os
import cv2
from PIL import Image, ImageDraw, ImageFont
import numpy as np
def create_video(text, font_path, font_size, output_path, fps=60, seconds=10, resolution=(1920, 1080), bg_color=(255, 0, 0), text_color=(255, 255, 255)):
# 获取文本宽度
font = ImageFont.truetype(font_path, font_size)
img = Image.new('RGB', (1, 1), color=(255, 255, 255))
draw = ImageDraw.Draw(img)
text_width, text_height = draw.textsize(text, font)
# 设置视频参数
width = resolution[0]
height = resolution[1]
frames = fps * seconds
# 创建视频
fourcc = cv2.VideoWriter_fourcc(*'mp4v')
video = cv2.VideoWriter(output_path, fourcc, fps, (width, height))
# 生成每一帧的图像
for i in range(frames):
# 创建背景
background = Image.new('RGB', (width, height), color=bg_color)
# 创建文本
text_image = Image.new('RGB', (text_width, text_height), color=bg_color)
draw = ImageDraw.Draw(text_image)
draw.text((0, 0), text, font=font, fill=text_color)
# 将文本贴到背景上
x_offset = (i - frames) * (text_width + 100) // frames
background.paste(text_image, (x_offset, (height-text_height)//2))
# 将图像转换为视频帧
frame = cv2.cvtColor(np.array(background), cv2.COLOR_RGB2BGR)
video.write(frame)
# 释放视频
video.release()
# 示例
text = "这是一个跑马灯视频"
font_path = "msyh.ttc"
font_size = 1000
output_path = os.path.join("E:\Template\word", "output.mp4")
fps = 60
seconds = 10
resolution = (1920, 1080)
bg_color = (255, 0, 0)
text_color = (255, 255, 255)
create_video(text, font_path, font_size, output_path, fps, seconds, resolution, bg_color, text_color)
阅读全文
相关推荐
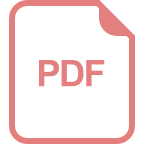
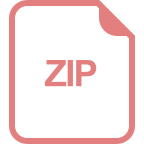
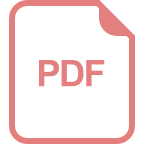















