wpf mvvm 基于 prism 包含model的示例
时间: 2023-10-10 21:06:27 浏览: 44
以下是一个基于Prism的WPF MVVM示例,其中包含模型:
首先,创建一个名为“Models”的文件夹,并在其中添加一个名为“Person.cs”的类:
```csharp
using System;
namespace PrismExample.Models
{
public class Person
{
public string FirstName { get; set; }
public string LastName { get; set; }
public int Age { get; set; }
public string Email { get; set; }
}
}
```
接下来,创建一个名为“ViewModels”的文件夹,并添加一个名为“MainViewModel.cs”的类。该类将作为我们的主界面的视图模型:
```csharp
using Prism.Commands;
using Prism.Mvvm;
using PrismExample.Models;
namespace PrismExample.ViewModels
{
public class MainViewModel : BindableBase
{
private Person _person;
public Person Person
{
get { return _person; }
set { SetProperty(ref _person, value); }
}
public DelegateCommand SaveCommand { get; private set; }
public MainViewModel()
{
Person = new Person();
SaveCommand = new DelegateCommand(Save);
}
private void Save()
{
// 保存Person信息的代码
}
}
}
```
最后,创建一个名为“Views”的文件夹,并在其中添加一个名为“MainWindow.xaml”的文件。在该文件中,我们将使用数据绑定将视图模型绑定到UI元素:
```xaml
<Window x:Class="PrismExample.Views.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:vm="clr-namespace:PrismExample.ViewModels"
Title="MainWindow" Height="350" Width="525">
<Window.DataContext>
<vm:MainViewModel />
</Window.DataContext>
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="Auto" />
<RowDefinition Height="Auto" />
<RowDefinition Height="Auto" />
<RowDefinition Height="Auto" />
<RowDefinition Height="Auto" />
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="Auto" />
<ColumnDefinition />
</Grid.ColumnDefinitions>
<Label Grid.Row="0" Grid.Column="0" Content="First Name:" />
<TextBox Grid.Row="0" Grid.Column="1" Text="{Binding Person.FirstName, UpdateSourceTrigger=PropertyChanged}" />
<Label Grid.Row="1" Grid.Column="0" Content="Last Name:" />
<TextBox Grid.Row="1" Grid.Column="1" Text="{Binding Person.LastName, UpdateSourceTrigger=PropertyChanged}" />
<Label Grid.Row="2" Grid.Column="0" Content="Age:" />
<TextBox Grid.Row="2" Grid.Column="1" Text="{Binding Person.Age, UpdateSourceTrigger=PropertyChanged}" />
<Label Grid.Row="3" Grid.Column="0" Content="Email:" />
<TextBox Grid.Row="3" Grid.Column="1" Text="{Binding Person.Email, UpdateSourceTrigger=PropertyChanged}" />
<Button Grid.Row="4" Grid.Column="1" Content="Save" Command="{Binding SaveCommand}" />
</Grid>
</Window>
```
现在,运行应用程序,并尝试在文本框中输入一些信息,然后单击“保存”按钮,即可成功保存Person信息。
相关推荐
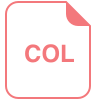
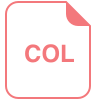
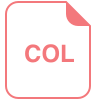
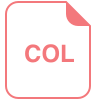
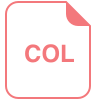









