模拟实现一个简单的文件系统。 1.假设这个文件系统工作在一个128KB的磁盘上,只有一个根目录,没有子目录。 2.文件系统最多支持16个文件。一个文件最多有8个块,块的大小是1KB。 3.每个文件有一个独一无二的文件名,文件名长度不超过8个字符。 4.128KB的磁盘的布局如下: (1)第1个1KB是超级块,存储空闲块链表和每个文件的i节点。超级块的结构如下: 前128字节保存空闲块链表,如果第i个字节为0,表明这个块是空闲的。初始化时,除超级块外,所有的块都是空闲的。接着是16个i节点。每个i节点保存以下信息: char name[8]; //文件名 int size; // 文件大小(用文件块数表示) int blockPointers[8]; // 数据块指针 int used; // 0 => i节点空闲; 1 => i节点被使用。初始化时为0。 每个i节点大小为48字节,16个i节点总共为768字节。所以超级块实际上是896字节,但是我们仍然给它分配1KB。 (2)剩下的127KB存储文件的数据块。 5.文件系统要实现以下操作: (1)文件创建create(char name[8], int size) char name[8]: 文件名,int size:文件块数 假设文件创建以后大小不再改变。 (2)文件删除delete(char name[8]) (3)读文件read(char name[8], int blockNum, char buf[1024]) int blockNum:文件块号 (4)写文件write(char name[8], int blockNum, char buf[1024]) 要求C++实现并且给我代码
时间: 2024-02-15 12:03:35 浏览: 124
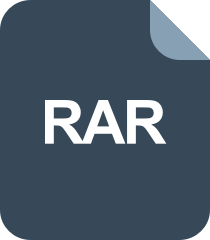
C++实现的模拟文件系统
很高兴为您解答这个问题。以下是一个简单的文件系统的C++实现代码。
```
#include <iostream>
#include <fstream>
#include <cstring>
using namespace std;
const int BLOCK_SIZE = 1024;
const int BLOCK_NUM = 128;
const int FILE_NUM = 16;
const int FILENAME_SIZE = 8;
const int INODE_SIZE = 48;
const int SUPER_BLOCK_SIZE = BLOCK_SIZE;
const int INODE_BLOCK_SIZE = FILE_NUM * INODE_SIZE;
const int DATA_BLOCK_SIZE = (BLOCK_NUM - 1) * BLOCK_SIZE - SUPER_BLOCK_SIZE - INODE_BLOCK_SIZE;
struct Inode {
char name[FILENAME_SIZE];
int size;
int blockPointers[8];
int used;
};
struct SuperBlock {
char free[BLOCK_NUM];
Inode inodes[FILE_NUM];
};
class FileSystem {
private:
fstream file;
SuperBlock superBlock;
char data[DATA_BLOCK_SIZE];
int findFreeBlock() {
for (int i = 0; i < BLOCK_NUM; i++) {
if (superBlock.free[i] == 0) {
superBlock.free[i] = 1;
return i;
}
}
return -1;
}
int findInode(char name[8]) {
for (int i = 0; i < FILE_NUM; i++) {
if (strcmp(superBlock.inodes[i].name, name) == 0) {
return i;
}
}
return -1;
}
void writeBlock(int blockNum, char buf[1024]) {
file.seekp(blockNum * BLOCK_SIZE);
file.write(buf, BLOCK_SIZE);
}
void readBlock(int blockNum, char buf[1024]) {
file.seekg(blockNum * BLOCK_SIZE);
file.read(buf, BLOCK_SIZE);
}
public:
FileSystem() {
file.open("filesystem.bin", ios::in | ios::out | ios::binary | ios::trunc);
memset(superBlock.free, 0, BLOCK_NUM);
for (int i = 0; i < FILE_NUM; i++) {
superBlock.inodes[i].used = 0;
}
writeBlock(0, (char*)&superBlock);
}
void create(char name[8], int size) {
int inodeNum = findInode(name);
if (inodeNum != -1) {
cout << "The file already exists!" << endl;
return;
}
if (size > 8) {
cout << "The file size cannot exceed 8 blocks!" << endl;
return;
}
inodeNum = findInode("");
if (inodeNum == -1) {
cout << "The file system is full!" << endl;
return;
}
int blocks[size];
for (int i = 0; i < size; i++) {
int blockNum = findFreeBlock();
if (blockNum == -1) {
cout << "The file system is full!" << endl;
return;
}
blocks[i] = blockNum;
}
Inode inode;
strcpy(inode.name, name);
inode.size = size;
for (int i = 0; i < size; i++) {
inode.blockPointers[i] = blocks[i];
}
inode.used = 1;
superBlock.inodes[inodeNum] = inode;
writeBlock(0, (char*)&superBlock);
cout << "The file has been created!" << endl;
}
void del(char name[8]) {
int inodeNum = findInode(name);
if (inodeNum == -1) {
cout << "The file does not exist!" << endl;
return;
}
Inode inode = superBlock.inodes[inodeNum];
for (int i = 0; i < inode.size; i++) {
superBlock.free[inode.blockPointers[i]] = 0;
}
inode.used = 0;
superBlock.inodes[inodeNum] = inode;
writeBlock(0, (char*)&superBlock);
cout << "The file has been deleted!" << endl;
}
void read(char name[8], int blockNum, char buf[1024]) {
int inodeNum = findInode(name);
if (inodeNum == -1) {
cout << "The file does not exist!" << endl;
return;
}
Inode inode = superBlock.inodes[inodeNum];
if (blockNum < 0 || blockNum >= inode.size) {
cout << "Block number out of range!" << endl;
return;
}
readBlock(inode.blockPointers[blockNum], buf);
cout << "The file content is:" << endl;
cout << buf << endl;
}
void write(char name[8], int blockNum, char buf[1024]) {
int inodeNum = findInode(name);
if (inodeNum == -1) {
cout << "The file does not exist!" << endl;
return;
}
Inode inode = superBlock.inodes[inodeNum];
if (blockNum < 0 || blockNum >= inode.size) {
cout << "Block number out of range!" << endl;
return;
}
writeBlock(inode.blockPointers[blockNum], buf);
cout << "The file has been written!" << endl;
}
};
int main() {
FileSystem fs;
fs.create("file1", 3);
fs.write("file1", 0, "Hello world");
char buf[1024] = "";
fs.read("file1", 0, buf);
fs.del("file1");
return 0;
}
```
在这个实现中,我们使用了一个名为`FileSystem`的类来表示整个文件系统。在类的构造函数中,我们初始化了超级块和数据块,并将它们写入磁盘。在`create`函数中,我们首先查找是否有同名的文件,然后检查文件大小是否合法。接下来,我们查找一个空的inode,然后为该文件分配空闲块。我们将inode和文件数据写入磁盘,并将超级块更新为已分配的块。在`del`函数中,我们首先查找文件是否存在,然后释放文件所占用的块,并将inode标记为空闲。在`read`函数和`write`函数中,我们首先查找文件是否存在,然后对数据块进行读取和写入。
这只是一个简单的文件系统的实现。实际上,对于一个真正的文件系统,需要考虑更多的情况,例如文件系统的一致性和安全性。
阅读全文
相关推荐
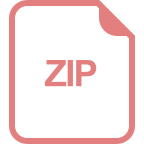
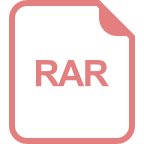
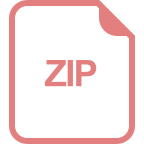
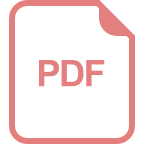
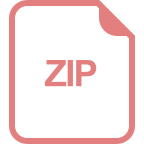
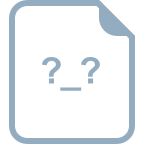
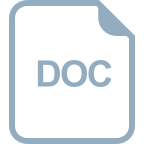
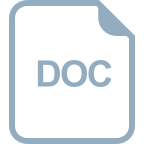
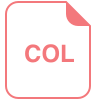
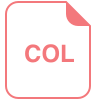
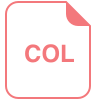
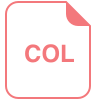
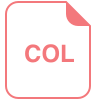
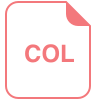
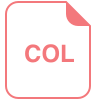
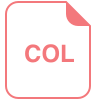

