c++ 找出std::map<std::string, int> myMap中最大的值所对应的键。
时间: 2024-09-19 10:16:49 浏览: 27
在 C++ 中,如果你想找出 `std::map<std::string, int>` 类型的数据结构 `myMap` 中,值最大的键,你需要遍历整个映射,并记住最大值及其对应的键。由于 C++ 没有内置的方法直接返回最大值对应的键,我们需要手动实现。以下是相应的代码片段:
```cpp
#include <iostream>
#include <map>
#include <string>
std::map<std::string, int> myMap; // 示例 map
void findMaxValueAndKey() {
if (myMap.empty()) {
std::cout << "Map is empty!" << std::endl;
return;
}
// 初始化最大值和对应的键
int maxValue = *myMap.begin()->second;
std::string maxKey = myMap.begin()->first;
// 遍历 map
for (const auto& entry : myMap) {
if (entry.second > maxValue) {
maxValue = entry.second;
maxKey = entry.first;
}
}
std::cout << "The key with the largest value is: " << maxKey << ", and the value is: " << maxValue << std::endl;
}
int main() {
// 填充 map
myMap["one"] = 10;
myMap["two"] = 20;
myMap["three"] = 30;
findMaxValueAndKey();
return 0;
}
```
这段代码首先检查 map 是否为空,然后设置最大值为第一个条目的值,键也为第一个键。然后它遍历整个 map,如果遇到值更大的项,就更新最大值和键。
相关推荐
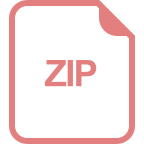
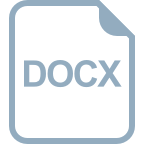
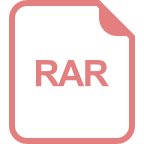















