写一个基于pytorch的脑电信号数据增强代码
时间: 2024-05-02 17:19:02 浏览: 142
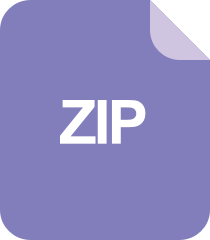
人工智能-项目实践-数据增强-pytorch实现MaxViT,可以在ImageNet或自己的数据集上训练,支持apex混合
以下是一个基于PyTorch的脑电信号数据增强代码示例:
```python
import torch
import numpy as np
from scipy import signal
class EEGAugmentation(torch.nn.Module):
def __init__(self, freq_range=[0.5, 16], time_shift_range=[-0.1, 0.1],
amplitude_range=[0.8, 1.2], noise_range=[-0.1, 0.1]):
super().__init__()
self.freq_range = freq_range
self.time_shift_range = time_shift_range
self.amplitude_range = amplitude_range
self.noise_range = noise_range
def forward(self, x):
x = self.time_shift(x)
x = self.change_frequency(x)
x = self.change_amplitude(x)
x = self.add_noise(x)
return x
def time_shift(self, x):
time_shift = np.random.uniform(
self.time_shift_range[0], self.time_shift_range[1])
num_samples = x.shape[-1]
time_shift_samples = int(time_shift * num_samples)
if time_shift_samples > 0:
x[:, :, time_shift_samples:] = x[:, :, :-time_shift_samples]
x[:, :, :time_shift_samples] = 0
elif time_shift_samples < 0:
x[:, :, :time_shift_samples] = x[:, :, -time_shift_samples:]
x[:, :, time_shift_samples:] = 0
return x
def change_frequency(self, x):
freq = np.random.uniform(
self.freq_range[0], self.freq_range[1])
b, a = signal.butter(4, freq, 'lowpass', fs=250)
for i in range(x.shape[0]):
for j in range(x.shape[1]):
x[i, j, :] = signal.filtfilt(b, a, x[i, j, :])
return x
def change_amplitude(self, x):
amplitude = np.random.uniform(
self.amplitude_range[0], self.amplitude_range[1])
x = x * amplitude
return x
def add_noise(self, x):
noise = np.random.uniform(
self.noise_range[0], self.noise_range[1], size=x.shape)
x = x + noise
return x
```
该代码实现了四种数据增强技术:
1. 时间偏移:随机平移信号的时间轴。
2. 频率变换:随机降低信号的频率,以模拟低通滤波器的效果。
3. 幅度变换:随机缩放信号的幅度。
4. 增加噪声:随机增加一些噪声。
可以使用如下方式来使用该数据增强模块:
```python
augmentation = EEGAugmentation()
x_augmented = augmentation(x)
```
其中 `x` 是一个三维张量,表示脑电信号数据。第一维表示样本数,第二维表示通道数,第三维表示时间序列长度。
阅读全文
相关推荐
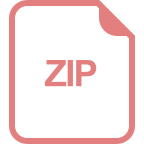
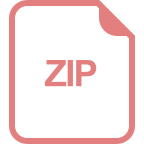
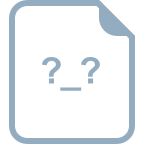
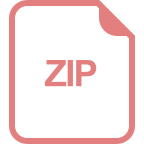


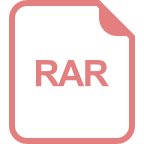
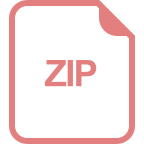
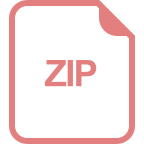
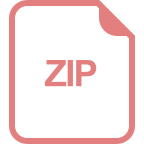
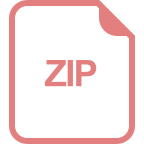
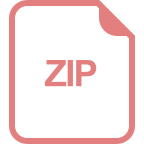
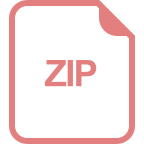
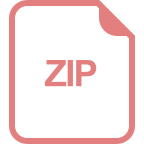
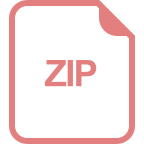