python 用灰度共生矩阵提取图像特征
时间: 2024-03-09 21:21:06 浏览: 23
灰度共生矩阵 (Gray-Level Co-occurrence Matrix, GLCM) 是一种描述图像纹理特征的方法,常用于图像分类、识别和分割等任务。它是基于图像中像素灰度值之间的空间关系来计算特征的。
下面是使用 Python 中的 skimage 库提取灰度共生矩阵的示例代码:
```python
from skimage.feature import greycomatrix, greycoprops
import numpy as np
from PIL import Image
# 读取图像并转为灰度图
img = Image.open('image.png').convert('L')
img_arr = np.array(img)
# 计算灰度共生矩阵
glcm = greycomatrix(img_arr, [1], [0, np.pi/4, np.pi/2, 3*np.pi/4])
# 计算灰度共生矩阵的特征
contrast = greycoprops(glcm, 'contrast')
dissimilarity = greycoprops(glcm, 'dissimilarity')
homogeneity = greycoprops(glcm, 'homogeneity')
energy = greycoprops(glcm, 'energy')
correlation = greycoprops(glcm, 'correlation')
# 输出特征值
print('Contrast:', contrast)
print('Dissimilarity:', dissimilarity)
print('Homogeneity:', homogeneity)
print('Energy:', energy)
print('Correlation:', correlation)
```
在上面的示例代码中,首先读取图像并转为灰度图,然后计算灰度共生矩阵。其中,`greycomatrix` 函数的第一个参数为输入图像的数组,第二个参数为灰度共生矩阵的距离(这里只计算距离为 1 的像素对),第三个参数为灰度共生矩阵的方向(这里计算 4 个方向)。接着,使用 `greycoprops` 函数计算灰度共生矩阵的特征,包括对比度(contrast)、不相似度(dissimilarity)、同质性(homogeneity)、能量(energy)和相关性(correlation)。最后输出特征值即可。
需要注意的是,灰度共生矩阵的计算依赖于图像的灰度级数,因此在使用 `greycomatrix` 函数时需要指定灰度级数,例如:
```python
glcm = greycomatrix(img_arr, [1], [0, np.pi/4, np.pi/2, 3*np.pi/4], levels=256)
```
其中,`levels` 参数指定灰度级数为 256。
相关推荐
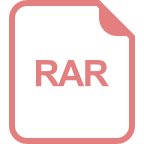













