请描述C语言代码bluez被其他设备配对的过程
时间: 2024-02-12 20:09:06 浏览: 142
在C语言中使用Bluez库实现蓝牙配对过程的基本步骤如下:
1. 初始化Bluez库:使用bluetooth/bluetooth.h头文件中的bluetooth_init()函数初始化Bluez库,获得一个bt_context对象。例如:
```
bt_context *context = NULL;
context = bluetooth_init();
if (context == NULL) {
printf("Failed to initialize Bluetooth library\n");
return -1;
}
```
2. 扫描设备:使用bluetooth/hci.h头文件中的hci_inquiry()函数扫描设备,该函数将发送一个Inquiry请求给周围的设备,并接收它们的响应。在响应中包含了设备的基本信息,如设备地址、设备名称等。例如:
```
inquiry_info **info = NULL;
int num_rsp = 0;
int flags = 0;
int len = 8;
info = (inquiry_info **)malloc(sizeof(inquiry_info *) * MAX_DEVICES);
num_rsp = hci_inquiry(0, len, MAX_DEVICES, NULL, &info, flags);
if (num_rsp < 0) {
printf("hci_inquiry() failed\n");
return -1;
}
```
3. 获取设备列表:扫描到设备后,使用bluetooth/hci.h头文件中的hci_get_remote_name()函数获取设备的名称和地址。例如:
```
for (int i = 0; i < num_rsp; ++i) {
char addr[19] = { 0 };
ba2str(&(info[i]->bdaddr), addr);
char name[248] = { 0 };
if (hci_get_remote_name(sock, &(info[i]->bdaddr), sizeof(name), name, 0) < 0) {
strcpy(name, "Unknown");
}
printf("%s %s\n", addr, name);
}
```
4. 建立连接:使用bluetooth/hci.h头文件中的hci_create_connection()函数建立与目标设备的连接。建立连接后,使用bluetooth/hci.h头文件中的hci_authenticate_link()函数发送配对请求。例如:
```
bdaddr_t addr;
str2ba(target_addr, &addr);
if (hci_create_connection(sock, &addr, 0x01, 0x01, 0, &err) < 0) {
printf("Failed to create connection to target device\n");
return -1;
}
if (hci_authenticate_link(sock, handle, 0) < 0) {
printf("Failed to send authenticate request\n");
return -1;
}
```
其中,target_addr为目标设备的蓝牙地址,handle为连接句柄。
5. 配对确认:目标设备接收到配对请求后,会生成一个PIN码或者是随机数,并将其发送给蓝牙设备。蓝牙设备收到PIN码或者是随机数后,会将其显示在屏幕上或者是提示用户输入。用户输入PIN码或者是确认随机数后,蓝牙设备会将其发送给目标设备进行确认。例如:
```
char pin[8] = { 0 }; // 用户输入的PIN码
if (hci_pin_code_request_reply(sock, &addr, 8, pin) < 0) {
printf("Failed to reply to PIN code request\n");
return -1;
}
```
需要注意的是,在实现蓝牙配对过程时,我们需要根据不同的配对方式选择相应的API函数。此外,在配对过程中还需要考虑到连接的安全性问题,比如密码认证等。具体实现时需要按照实际需求选择相应的安全措施。
阅读全文
相关推荐
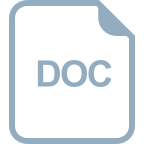
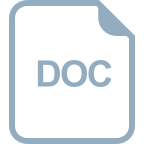
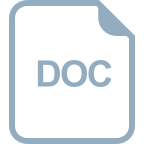


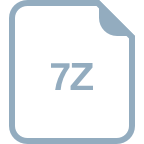
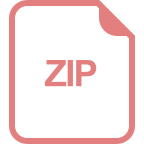
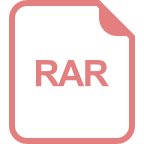
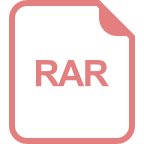
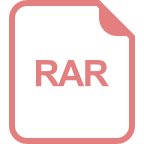
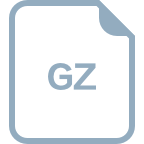
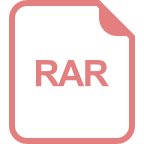
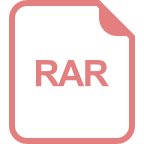
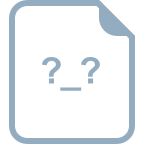
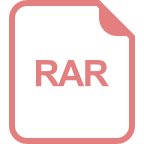
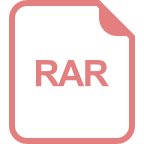
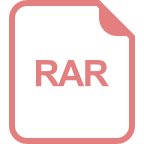
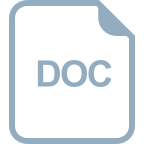
