如何在下列代码中减小 Adam 优化器的学习率(lr),以防止步长过大;以及在模型中增加 Batch Normalization 层,以确保模型更稳定地收敛;class MLP(torch.nn.Module): def init(self, weight_decay=0.01): super(MLP, self).init() self.fc1 = torch.nn.Linear(178, 100) self.relu = torch.nn.ReLU() self.fc2 = torch.nn.Linear(100, 50) self.fc3 = torch.nn.Linear(50, 5) self.dropout = torch.nn.Dropout(p=0.1) self.weight_decay = weight_decay def forward(self, x): x = self.fc1(x) x = self.relu(x) x = self.fc2(x) x = self.relu(x) x = self.fc3(x) return x def regularization_loss(self): reg_loss = torch.tensor(0.).to(device) for name, param in self.named_parameters(): if 'weight' in name: reg_loss += self.weight_decay * torch.norm(param) return reg_lossmodel = MLP() criterion = torch.nn.CrossEntropyLoss() optimizer = torch.optim.Adam(model.parameters(), lr=0.001) for epoch in range(num_epochs): for i, (inputs, labels) in enumerate(train_loader): optimizer.zero_grad() outputs = model(inputs.to(device)) loss = criterion(outputs, labels.to(device)) loss += model.regularization_loss() loss.backward() optimizer.step()
时间: 2023-06-06 16:06:39 浏览: 182
要减小Adam 优化器的学习率(lr),可以通过设置optimizer的参数lr来实现:optimizer = torch.optim.Adam(model.parameters(), lr=0.0001)。要在模型中增加 Batch Normalization 层以确保模型更稳定地收敛,可以在每个线性层(torch.nn.Linear)之后添加BatchNorm1d层(torch.nn.BatchNorm1d): class MLP(torch.nn.Module): def __init__(self, weight_decay=0.01): super(MLP, self).__init__() self.fc1 = torch.nn.Linear(178, 100) self.bn1 = torch.nn.BatchNorm1d(100) self.relu = torch.nn.ReLU() self.fc2 = torch.nn.Linear(100, 50) self.bn2 = torch.nn.BatchNorm1d(50) self.fc3 = torch.nn.Linear(50, 5) self.dropout = torch.nn.Dropout(p=0.1) self.weight_decay = weight_decay def forward(self, x): x = self.fc1(x) x = self.bn1(x) x = self.relu(x) x = self.fc2(x) x = self.bn2(x) x = self.relu(x) x = self.fc3(x) return x def regularization_loss(self): reg_loss = torch.tensor(0.).to(device) for name, param in self.named_parameters(): if 'weight' in name: reg_loss += self.weight_decay * torch.norm(param) return reg_loss model = MLP() criterion = torch.nn.CrossEntropyLoss() optimizer = torch.optim.Adam(model.parameters(), lr=0.0001) for epoch in range(num_epochs): for i, (inputs, labels) in enumerate(train_loader): optimizer.zero_grad() outputs = model(inputs.to(device)) loss = criterion(outputs, labels.to(device)) loss += model.regularization_loss() loss.backward() optimizer.step()
阅读全文
相关推荐
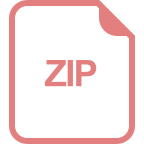
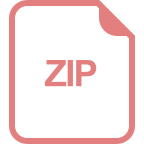
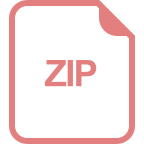
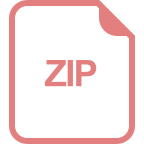
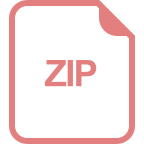
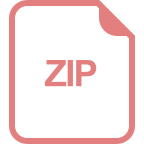
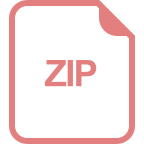
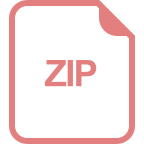
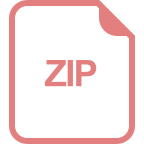
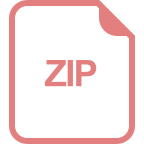
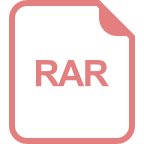