编程实现:编写程序实现一个简单的二进制位查看器,要求程序运行时从键盘输入一个double浮点数,将其在内存中的二进制位形式输出出来
时间: 2024-10-07 16:04:58 浏览: 43
在Java中,由于double类型的内部存储机制,我们不能直接获取其二进制比特流,只能得到它的近似二进制表示。以下是使用`IEEE 754`标准进行近似的输出,这个方法会提供一个64位的表示:
```java
import java.util.Scanner;
public class BinaryViewer {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter a double value:");
double input = scanner.nextDouble();
// Convert the double to hexadecimal and then back to binary (since Java doesn't have direct access)
String hex = Long.toHexString(Double.doubleToLongBits(input));
String binary = "";
for (char c : hex.toCharArray()) {
if (Character.isDigit(c)) {
binary += c;
} else if (c == 'p') {
binary += 'e'; // Replace 'p' with 'e'
} else if (c == '-') {
continue; // Skip negative sign since it's not part of the bit pattern
} else {
throw new IllegalArgumentException("Invalid character found");
}
}
// Display the binary representation
System.out.printf("Approximate binary representation of %.2f is %s\n", input, binary);
}
}
```
这个程序会提示用户输入一个double,然后输出一个近似的二进制表示。然而,需要注意的是,对于某些特定的非常小或非常大的double值,这个转换可能会失去精度。
阅读全文
相关推荐
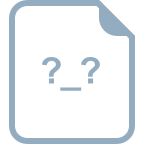
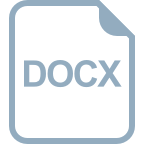
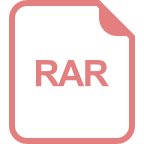
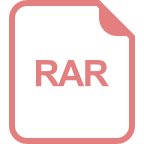
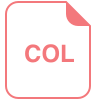
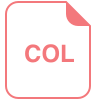
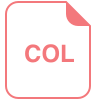
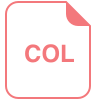
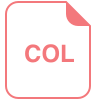
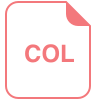
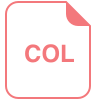
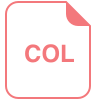
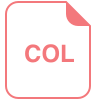
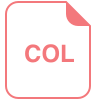
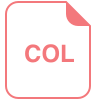
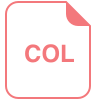
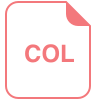
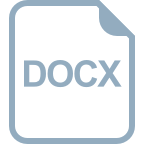
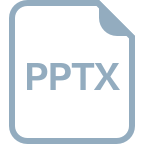