1)对输入数据构造成一棵Huffman 树; 2)对生成Huffman 树进行Huffman 编码c++代码
时间: 2024-02-15 21:01:18 浏览: 54
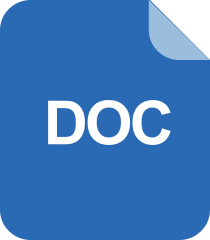
武汉理工大学算法与数据结构综合实验Huffman编码压缩存储.doc
1)构造Huffman树的代码:
```c++
#include <queue>
#include <vector>
struct Node {
int weight;
Node* left;
Node* right;
Node(int w, Node* l = nullptr, Node* r = nullptr) :
weight(w), left(l), right(r) {}
};
struct cmp {
bool operator()(const Node* lhs, const Node* rhs) const { return lhs->weight > rhs->weight; }
};
Node* constructHuffmanTree(const std::vector<int>& weights) {
std::priority_queue<Node*, std::vector<Node*>, cmp> pq;
for (auto w : weights) {
pq.push(new Node(w));
}
while (pq.size() > 1) {
auto left = pq.top();
pq.pop();
auto right = pq.top();
pq.pop();
auto parent = new Node(left->weight + right->weight, left, right);
pq.push(parent);
}
return pq.top();
}
```
2)生成Huffman编码的代码:
```c++
#include <unordered_map>
#include <string>
void generateHuffmanCode(Node* root, const std::string& prefix, std::unordered_map<char, std::string>& codeMap) {
if (!root) return;
if (!root->left && !root->right) {
codeMap.insert({ prefix[0], prefix.substr(1) });
return;
}
generateHuffmanCode(root->left, prefix + "0", codeMap);
generateHuffmanCode(root->right, prefix + "1", codeMap);
}
std::unordered_map<char, std::string> getHuffmanCode(Node* root) {
std::unordered_map<char, std::string> codeMap;
generateHuffmanCode(root, "", codeMap);
return codeMap;
}
```
其中,`root`是Huffman树的根节点,`codeMap`是字符和对应编码的映射表。函数`generateHuffmanCode`递归地遍历Huffman树,对于每个叶子节点,记录下字符和对应的编码前缀,最终将映射表存入`codeMap`中。函数`getHuffmanCode`是对外暴露的接口,返回字符和对应编码的映射表。
阅读全文